Finally, a complete program to build complex networked apps in SwiftUI with a scalable architecture and code that is easy to read, test, and maintain
Get past material for beginners and trivial examples and learn the advanced techniques and practices I use to build apps for big clients with a network layer architecture that stands the test of time.
Including:
- Detailed coverage of Internet protocols: TCP/IP, DNS, HTTP, REST, TLS, and OAuth
- In-depth analysis of design patterns: MVC, MVVM, MV, and other variations
- The SOLID design principles for modular and reusable code
- Concurrency in Swift and SwiftUI: Multithreading, async/await, structured concurrency, and actors
- Network layer architecture with Protocol-oriented Programming and Swift generics
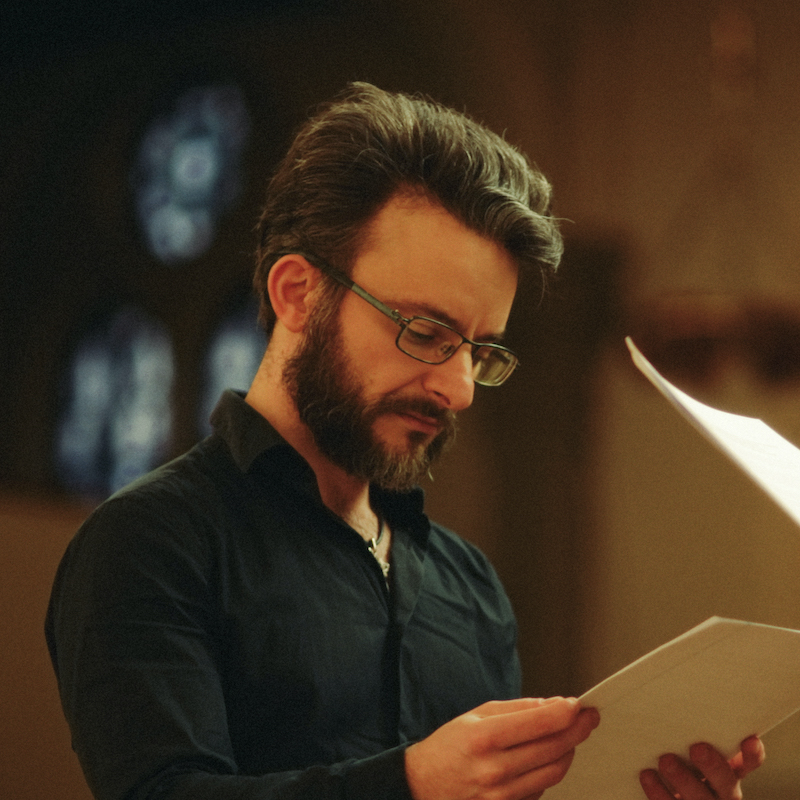
Hi, I’m Matteo Manferdini. For the past two decades, I have created iOS apps for big companies and published them in the App Store for iOS, iPadOS, and macOS.
Today, I teach iOS and SwiftUI development to 13,000+ subscribers, 6,000+ followers, and hundreds of students who have taken my courses.
In the past years, I have built complex iOS apps for large clients, working in large teams or as a solo developer. I have also charged high rates for my freelance work.
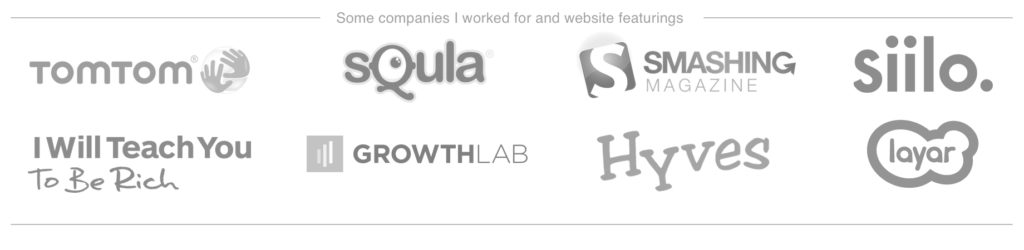
Building complex networked apps is an essential skill for every developer.
If you open the App Store, you’ll see that most of the top apps promoted by Apple connect to Internet servers.
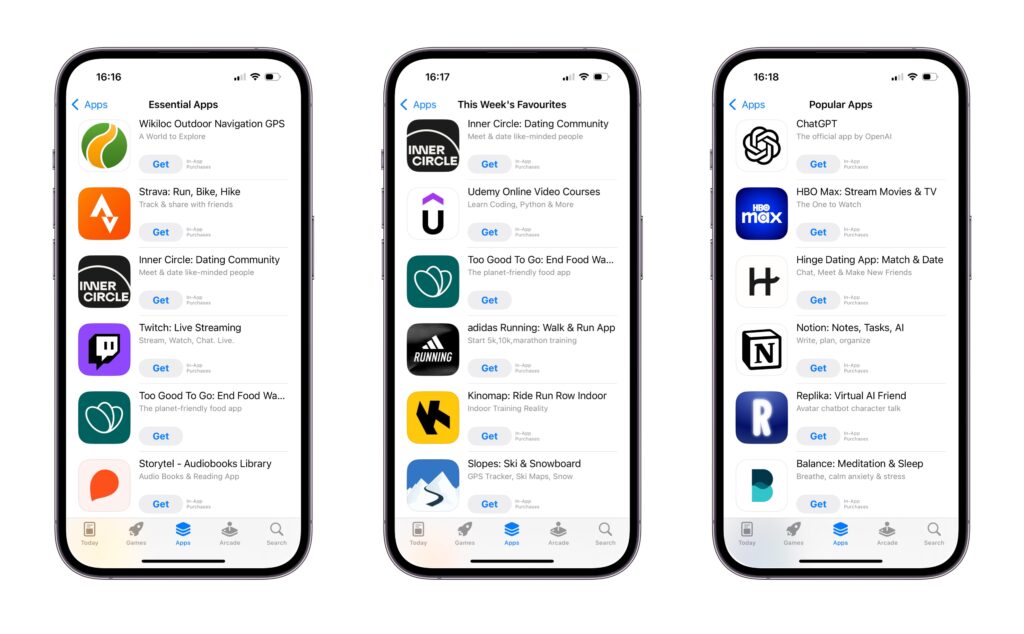
However, networked apps are not straightforward to make and stretch your development skills to the limit.
SwiftUI has made putting together an app as simple as ever. However, adding networking to your codebase can suddenly make its architecture overwhelming.
Initially, you can search on Google for networking tutorials and slap some code together. But soon, you get bitten by the complexity of making several asynchronous network calls to a remote REST API.
You cannot just copy the simple code samples of online tutorials into a real-world app. You must handle HTTP methods, headers, status codes, and authentication. Most modern REST APIs use OAuth, which adds even more complexity.
As you add networking code, you inevitably end with a massive network manager that devours all networking code like a black hole, making your code hard to understand and completely untestable.
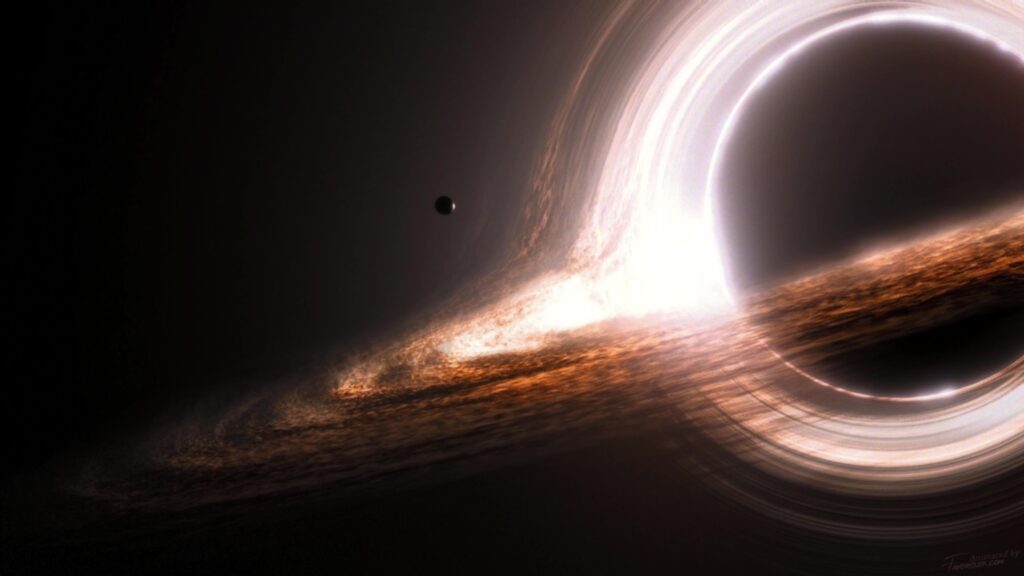
But how should you architect the networking layer of your app? Where should you place your networking code? Is using a network manager even a good idea?
And what about the whole app architecture? Should you use design patterns like MVC and MVVM, or is there something better for networked apps?
It’s hard to judge if the architectural approaches you find online are suitable. How are you supposed to know? Should you just trust a random blog post?
Nobody teaches these skills, so you are left wondering how to proceed once you pass the beginner stage.
Most blogs only show small code examples and do not discuss best practices. Architecture and design principles are rarely covered, and you can’t easily copy them from a Stack Overflow post.
Moreover, the majority of courses are geared toward entry-level developers. Many authors lack the experience to teach advanced topics and inadvertently pass poor practices as the norm.
Bad architecture can kill your project before it has a chance to get to the App Store
During my career, I have seen many projects that failed because of poor architectural choices.
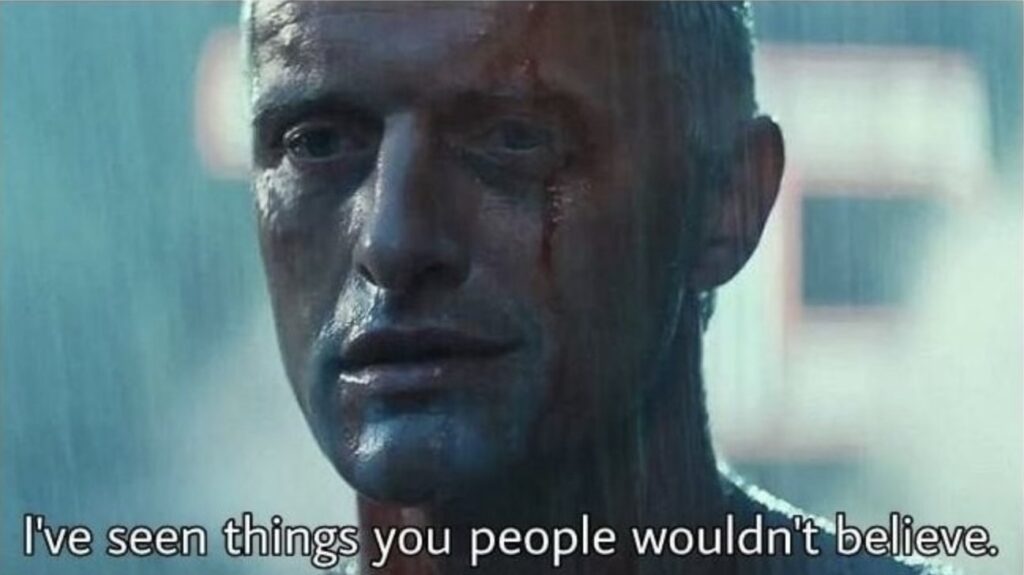
Years ago, I was hired by a small startup to fix the bugs in an app they were developing for a client.
As I started working on it, I realized the issues were more than bugs. Things rarely worked properly, and I was surprised they considered the app almost finished.
The app had a screen where users could create new items, but weird duplicates often appeared. Digging deeper, I discovered that the app sent duplicate POST requests to their REST API.
However, the problem could not be pinpointed to a single source. It was caused by the app’s architecture, how different parts communicated, and how objects reacted to events.
The causes were so widespread that a simple fix was impossible. When I tried patching the problem with quick workarounds to get the app to a releasable state, other issues would arise because of my fixes.
Ultimately, the entire codebase was thrown away, and another company stepped in to rewrite the app from scratch, consuming the entire budget and leaving no profit.
Complexity can affect even large companies with experienced developers
It would be wrong to think that these issues happen only in startups.
A few years ago, I worked for a large, renowned company with an app that was three years late on its deadline. The app was so crucial that even Apple pressured the company to finish it.
This was not a startup. The iOS team had more than 20 experienced developers, and I was hired again to fix bugs and speed up the release.
This time, the situation was better. The bugs were fixable, often with a simple change. However, finding what caused each bug and the proper fix took me days.
Whenever I explored the underlying architecture, I was confronted by an utterly tangled mess of classes that referenced each other and communicated indirectly through a custom reactive system developed in-house.
I tried drawing the class diagram on paper, but it didn’t help. It was too complicated.
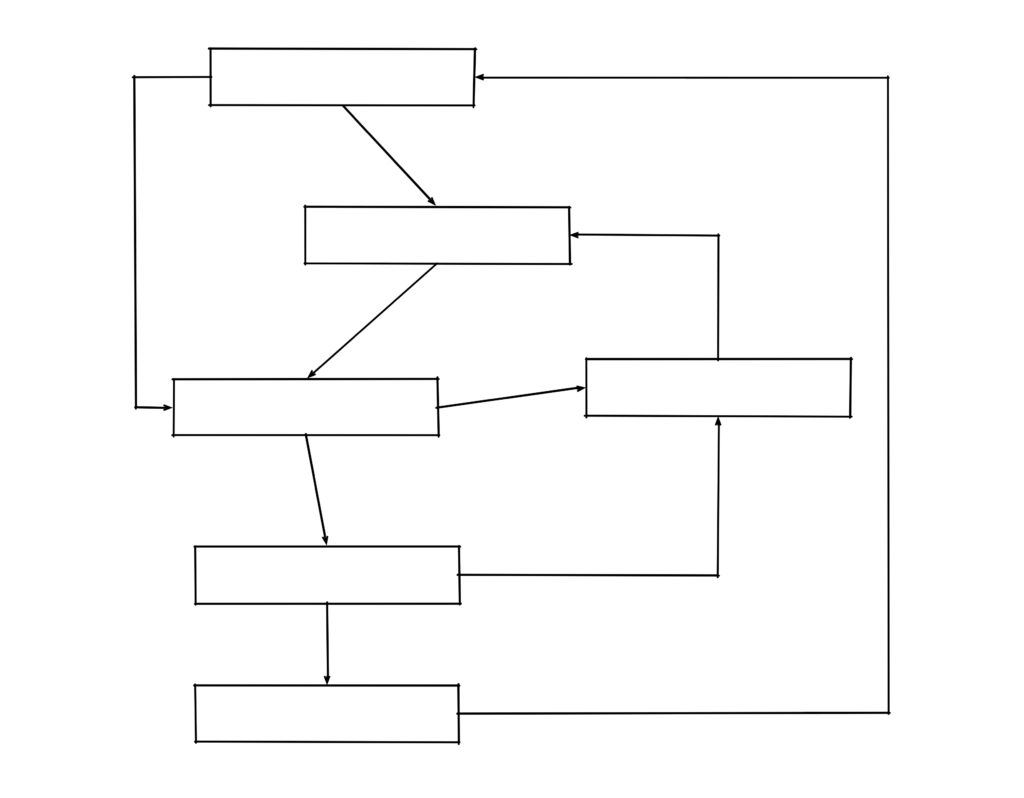
I made the same mistakes, and you probably do too
It’s easy to blame others, but I am guilty of the same crimes.
Before becoming a freelancer, I worked at an agency in Amsterdam, building an app for a large investment bank.
Because the app was connected to sensitive financial information, it had strict requirements and a complex authentication flow based on one-time passcodes, which was even more complicated than OAuth.
Because of the strict requirements, when I finished the app, the bank sent my code to a third party for review.
At that point in my career, I had become complacent. I thought I knew everything about iOS development. I produced well-written code, and my skills would impress the reviewers.
The verdict was not what I expected.
Their report was ruthless:
My code was too complicated and hard to maintain. Moreover, adding unit tests that ensured its correctness was impossible.
My arrogance prevented me from learning from that feedback. I felt the reviewers were not good enough to realize how great my code was. And who needs tests anyway? Nobody did that in iOS development.
That was 2012, so I could afford to know so little. With those skills, I wouldn’t go far in today’s job market.
Luckily, that blindness did not last long.
Not much later, I joined newsletters about iOS development and started reading articles about architecture and design patterns. My world shattered, and I realized how little I knew.
Ten years later, I can finally produce high-quality code that I am proud of
Looking back at when I thought I knew everything, I can now see all my shortcomings. In all honesty, I didn’t know much at all.
Since then, I have learned a lot. That’s why, when freelancing, I was hired by big companies with skilled developers.
I can now refactor code that would be impossible to test and make it testable.
I can see the shortcomings of existing code and its structural issues and then discuss the best course of action to fix them with other developers in a team.
I can read articles and books about new ideas and judge whether they are valid or where they could create problems.
I can do all this because I learned some significant insights about architecture.
Insight #1: Programming paradigms, development principles, and design patterns all work in concert with the architecture of a networked app
Reading many articles about iOS development makes it easy to get lost in buzzwords.
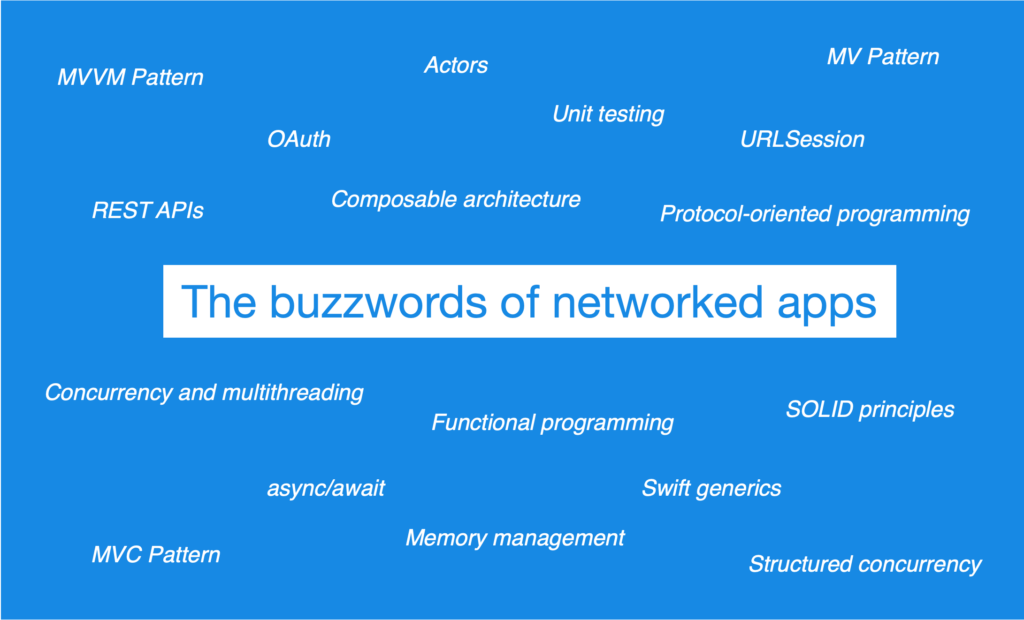
Which of these concepts should you use?
Protocol-oriented programming seems hot in Swift and is extensively used by SwiftUI. At WWDC2015, Apple declared, “Start with protocols first.” But does that also apply to networking code?
Moreover, some popular developers seem to advocate functional programming. How does that fit in SwiftUI? Doesn’t it contradict protocol-oriented programming?
What about the SOLID principles? Many repeat that they are essential, but aren’t they about object-oriented programming? How do they fit in SwiftUI apps?
The reality is that these concepts are all useful!
So, how do you use them all correctly in your apps?
The key is to understand that their purpose is the same. This means they overlap in many ways and approach the same problems from different angles.
They don’t contradict but complement each other.
Once you understand how these concepts work together, it’s easier to see how they relate to implementing a real app.
Many of my students are always surprised at how I can come up with clean solutions like these:
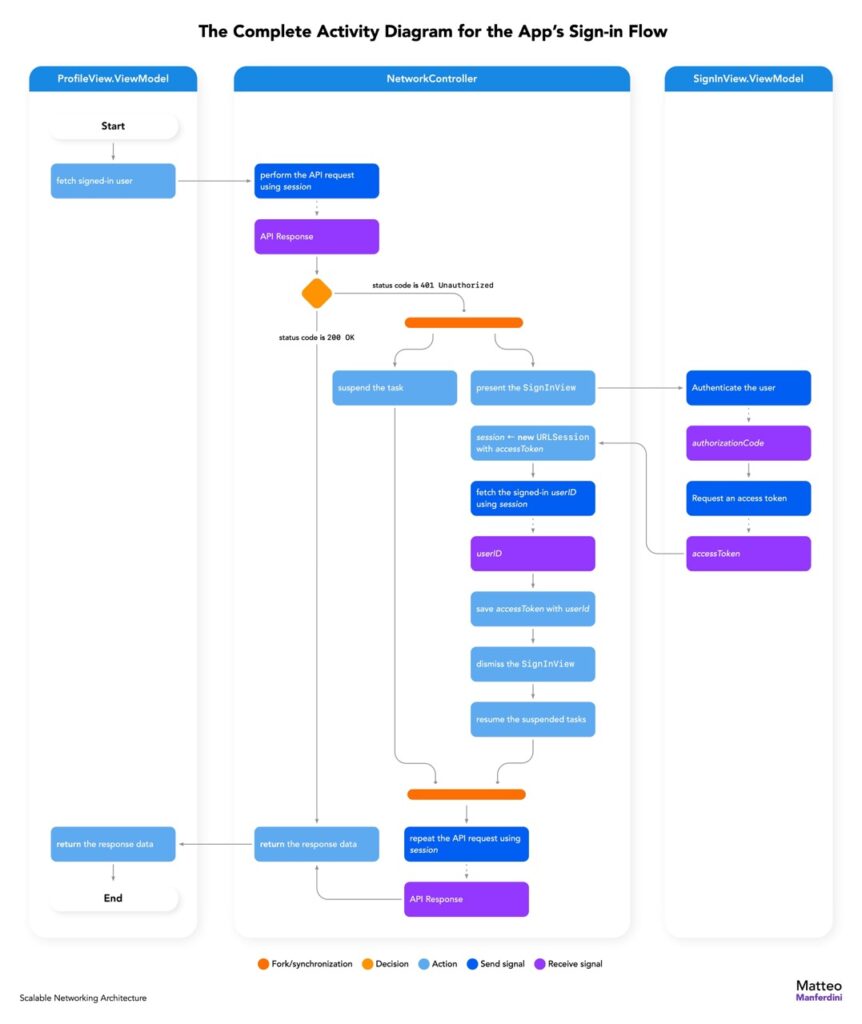
The truth is, I am no genius.
I know how to separate code because I understand how all concepts work together. I have also seen how these concepts work in practice and the problems that occur when ignored.
Insight #2: Good architecture comes from high-level design principles before focusing on low-level details
When developing SwiftUI apps, there is a tendency to focus on low-level implementation details.
This is a natural result of how we learn and the endless online examples that only focus on such details, disregarding software design principles and architectural design patterns.
Online, you can find endless tutorials and books about the fine-grained details of basic networking geared toward beginners, who make up most of the market.
Stack Overflow answers also only focus on details. Asking high-level questions about architecture is forbidden by the site guidelines.
I have learned that to develop a well-architected app, you must follow higher-level design principles before getting bogged down in details.
Your initial approach does not always have to be perfect. However, a solid initial structure lets you get the most important things right first and then focus on the low-level implementation details.
That’s not to say that the details are irrelevant. After all, they are what makes the app work. However, a project with the wrong architecture will eventually collapse under its weight.
App architecture is like a master painting. It starts with the large structure and design principles, which get progressively refined as you add the specific code that implements the app’s features.
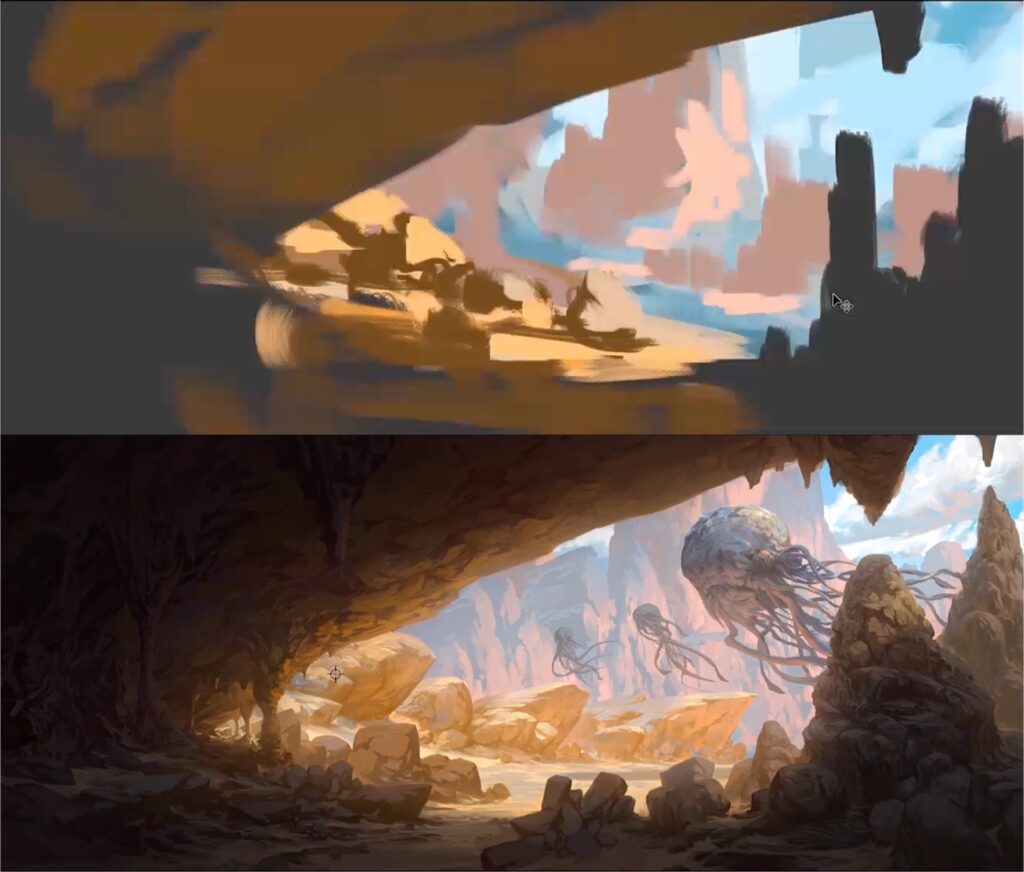
The great thing about code is that it can constantly be refactored. So, even if you make mistakes or have a codebase that seems unsalvageable, following the correct principles can help you turn your project around.
Insight #3: The correct architectural design patterns allow you to apply design principles without effort
It can be overwhelming to constantly think about programming paradigms, development principles, and design patterns.
But you don’t have to.
Architectural design patterns help enforce many best practices without constantly stressing. This was a great revelation for me.
It all starts with the MVC pattern.
Many developers think that MVC is not a very sophisticated pattern. While it is true that following its vanilla version can lead to problems like massive SwiftUI views or network managers, that is already better than nothing.
But MVC can be expanded. In a multilayer architecture, you can distribute responsibilities across more layers.
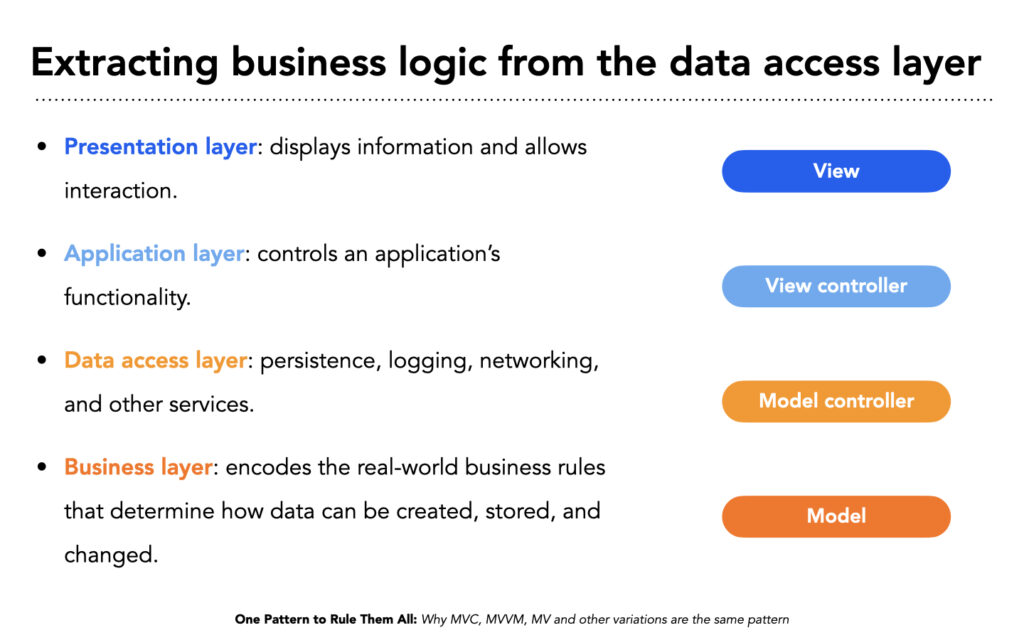
In SwiftUI, we can even introduce a fifth layer containing the responsibilities that SwiftUI strictly requires.
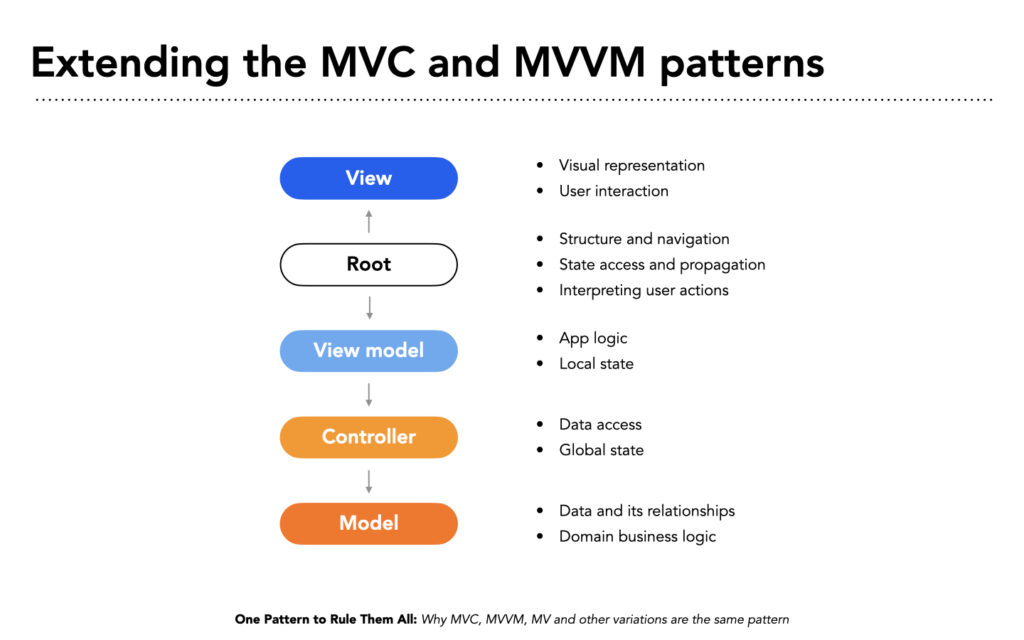
This helps push all networking code into the lower architectural layers, where it belongs, leading to a better separation of responsibilities. Moreover, unlike SwiftUI views, the resulting modular types are testable.
But there’s more.
Notice how the arrows in the diagram radiate from the root layer.
When examined closely, the SOLID principles encourage a top-down design of Swift types. However, that is not evident at first sight.
The tree structure of the MVC pattern for SwiftUI does that seamlessly, even if you don’t have a deep understanding of design principles.
An object graph with no cycles makes memory management with ARC much more straightforward, avoiding memory leaks. You can apply the same insight inside each architectural layer, making it easier to follow the execution flow of your code.
The cherry on the cake is that this fits the model of structured concurrency in Swift perfectly.
It took me a long time to gain these insights.
The information in online articles and books is too fragmented.
A book on Swift concurrency provides only a few techniques. How do they relate to developing a complex networked app? Specialized books usually don’t explain this.
You can also find more general books on high-level principles. A book often recommended is “Structure and Interpretation of Computer Programs.” You can even read it for free online.
It’s a book that has many great ideas. However, it was written in 1985, and all the code is in Lisp, a functional language entirely different from Swift. You need to learn a new language and an entire programming paradigm you will never use just to read that book.
And after that, how do you map the concepts back to SwiftUI? How do you know which are relevant and which do not belong to SwiftUI apps?
It took me years of study, experimentation, and discussion with other developers to combine these concepts into a coherent system.
Now, I want to share that system with you.
Introducing…
Scalable Networking Architecture
The complete SwiftUI networking course for iOS developers who feel stuck and want to go past simple online tutorials for beginners, moving from intermediate to expert.
Scalable Networking Architecture gives you the experience of a real-world project and teaches you the solutions to many practical software design problems.
It shows how to achieve a clean architecture and structure a large project’s code cleanly and professionally.
I put together many lessons and principles I learned in almost 20 years of working on Apple platforms in a unique system.
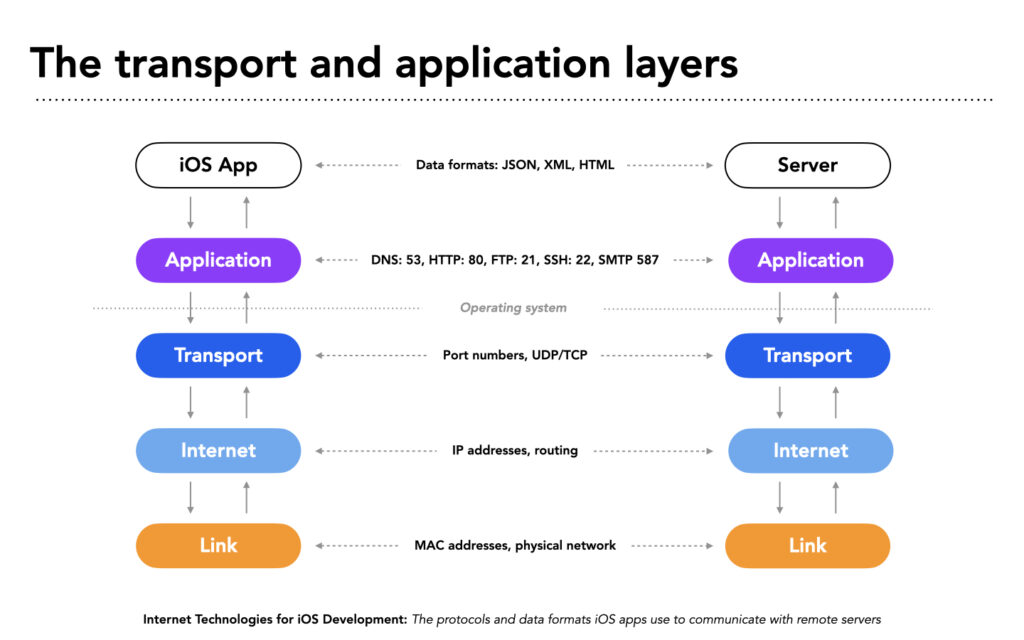
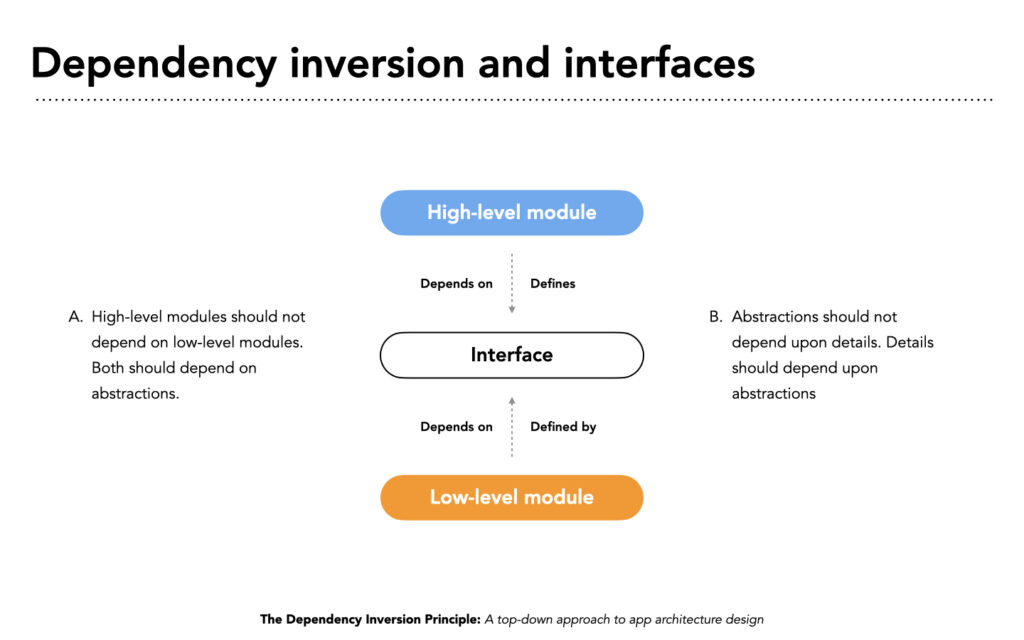
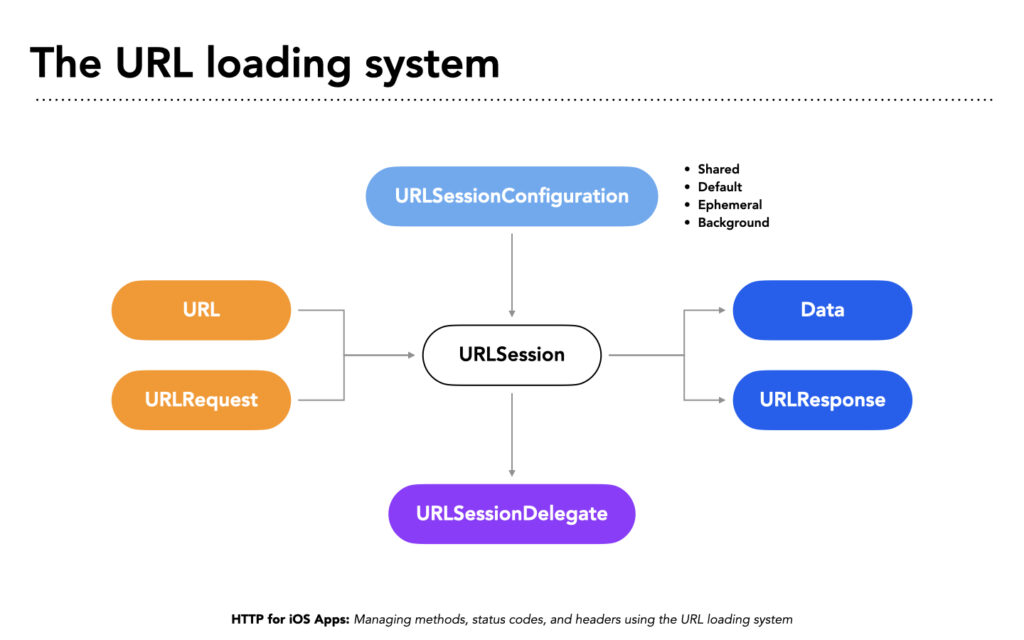
- Become an experienced developer who can build complex networked apps. Learn real-world techniques and practices for creating a clean architecture with well-organized modular components that are easy to understand and navigate.
- Fill in the missing pieces. Networking is not just about code. Architecting a fully networked app requires knowing Internet protocols like HTTP, server models like REST, and secure authentication with TLS and OAuth.
- Get out of tutorial hell. Move past the beginner stage and leave aside courses for entry-level developers. Watch how real developers work and think and learn how they design elegant code.
- Experience real-world scenarios. Deeply understand the ins and outs of multithreading, asynchronous functions, structured concurrency, actors, and how to address the strict concurrency checking of Swift 6.
- Follow a complete system that goes deeper and shows you the theory and the real-world practices to move your development forward with significant strides.
How Scalable Networking Architecture works
Scalable Networking Architecture is an online course that distills everything I have learned about creating a maintainable architecture for Internet-connected iOS apps in SwiftUI.
The course is divided into modules covering a specific piece of the whole system, going from Internet protocols and software design principles to architectural patterns, complex networking, Swift concurrency, and much more.
The course currently contains four modules, each with theoretical video lessons and practical hands-on lessons that explore in depth the practice of building networked SwiftUI apps with a scalable network layer.
More modules are under development and will be added in the coming months.
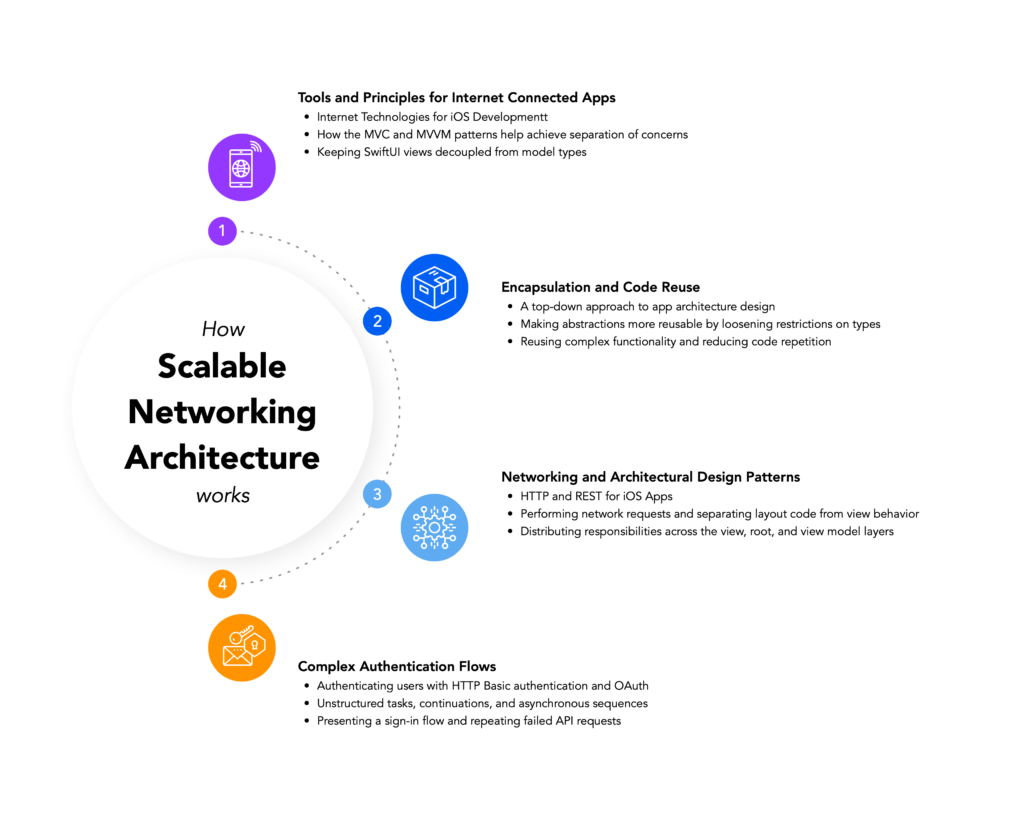
Inside the Scalable Networking Architecture system
Module 1
Tools and Principles for Internet-connected Apps
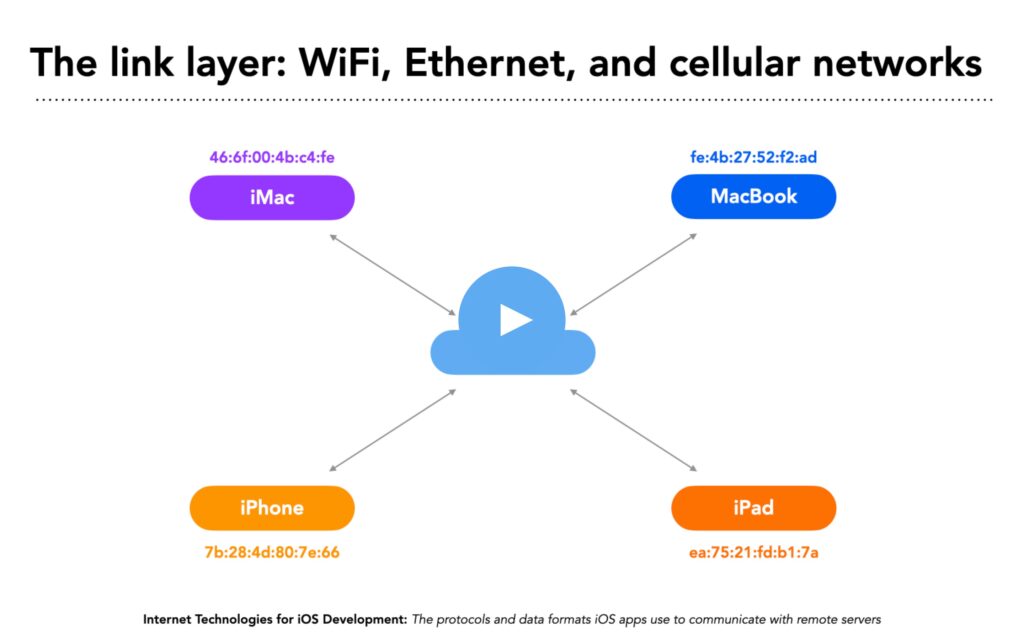
Lesson 1.1 – Internet Technologies for iOS Development
The protocols and data formats iOS apps use to communicate with remote servers
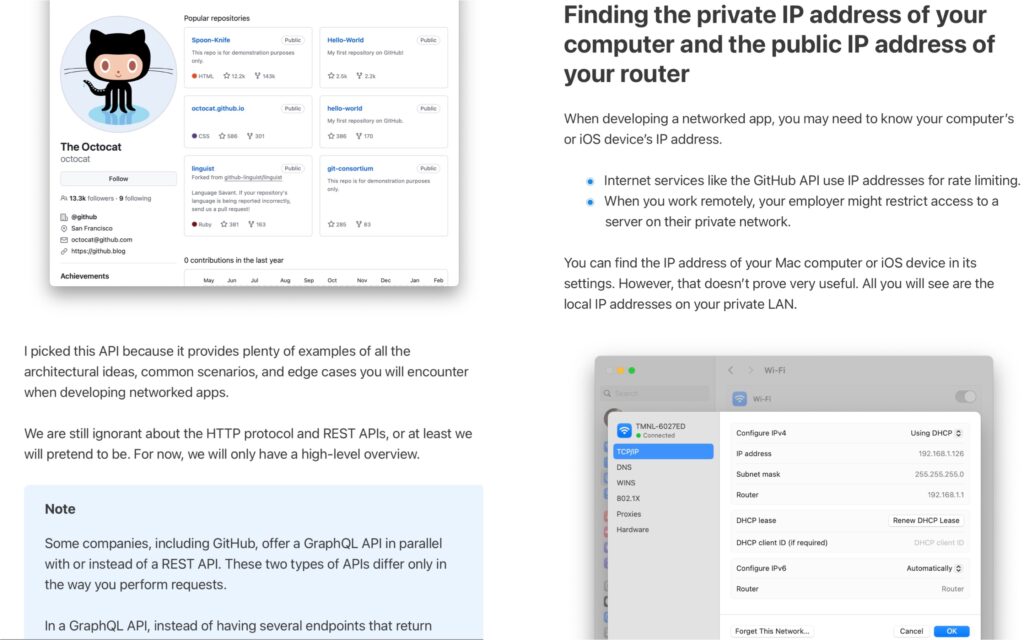
Lesson 1.2 – Documentation and Tools
Understanding how a REST API works and testing the network connection to the server
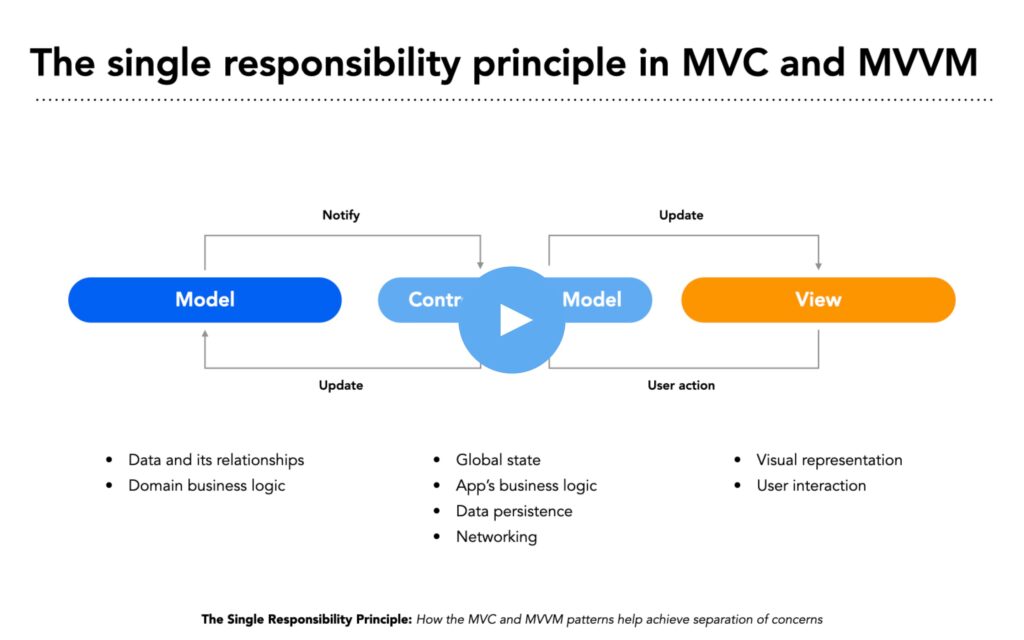
Lesson 1.3 – The Single-Responsibility Principle
How the MVC and MVVM patterns help achieve separation of concerns
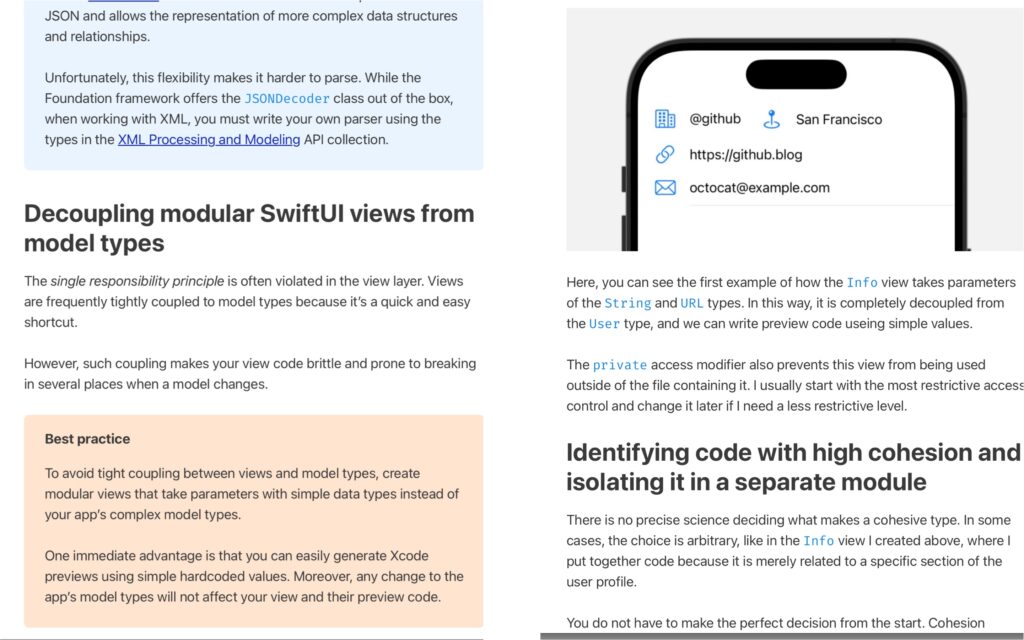
Lesson 1.4 – Models and Views
Decoding the data of a REST API and keeping SwiftUI views decoupled from model types
What you’ll learn:
- The Single-responsibility principle: how the MVC pattern effortlessly organizes your code and creates well-designed Swift types that are easy to reuse and straightforward to test, with more expressive and readable code.
- The structure of the Internet: how data moves across protocols and layers and reaches servers on far-away networks. Get the crucial concepts to build networked apps even if you don’t yet know how the Internet works.
- Isolating code with high cohesion to create modular SwiftUI views decoupled from the app’s model types. This foundational concept influences the entire architecture of a well-structured app.
- Using web browsers, HTTP, and command-line tools to test reachability and other common network problems. If you don’t know these simple tools, you won’t be able to troubleshoot your app’s networking issues.
- How to explore a REST API’s documentation and decode sample JSON data to build your app’s interface. Plus, the techniques to ensure Xcode previews always work without an internet connection.
Module 2
Encapsulation and Code Reuse
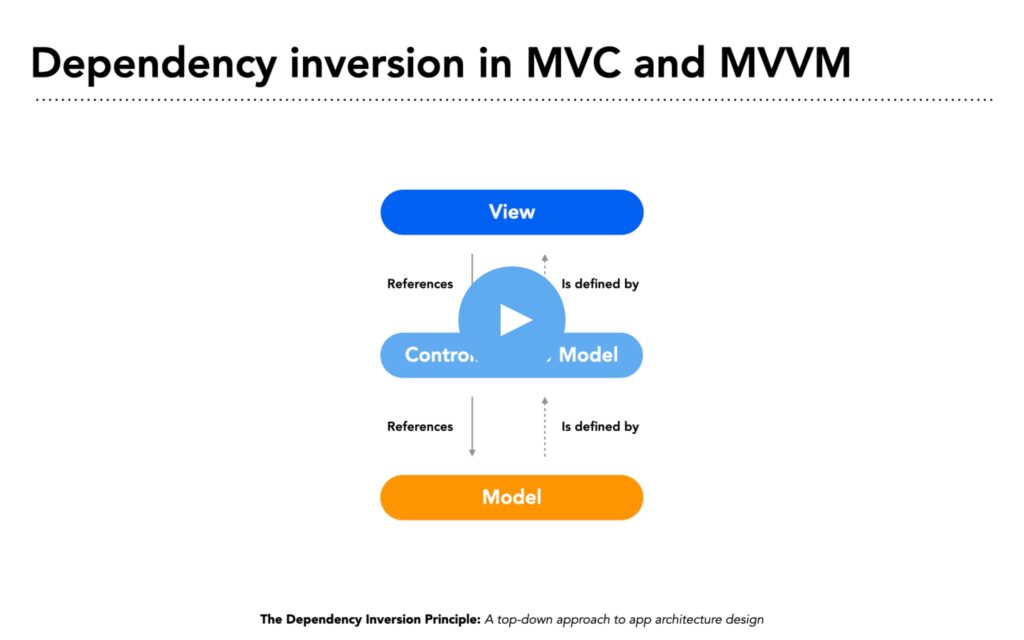
Lesson 2.1 – The Dependency Inversion Principle
A top-down approach to app architecture design
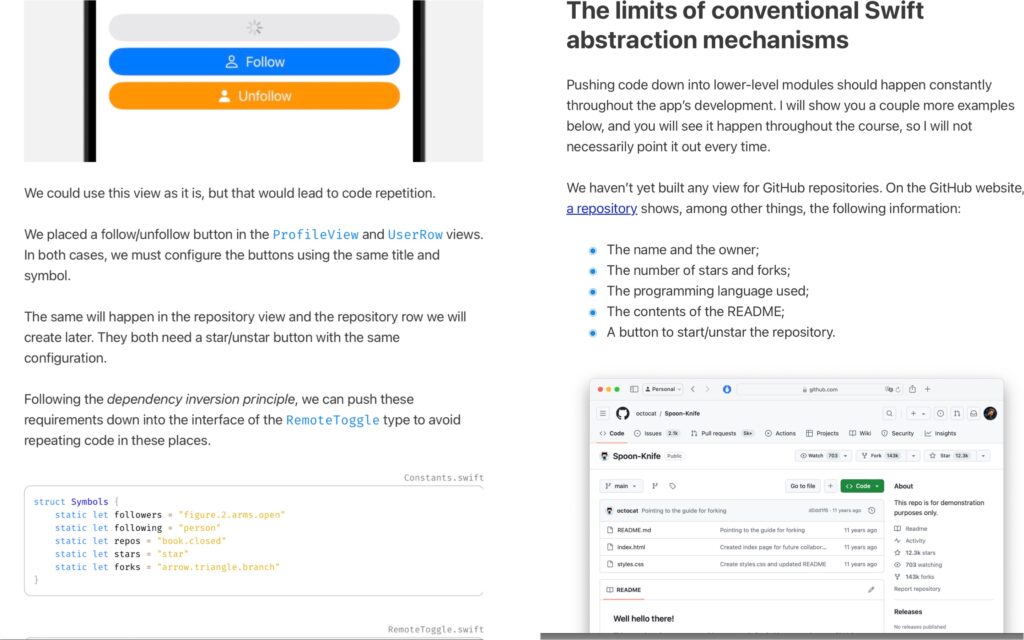
Lesson 2.2 – The Downward Pressure of SwiftUI Views
How high-level features influence the design of lower-level types
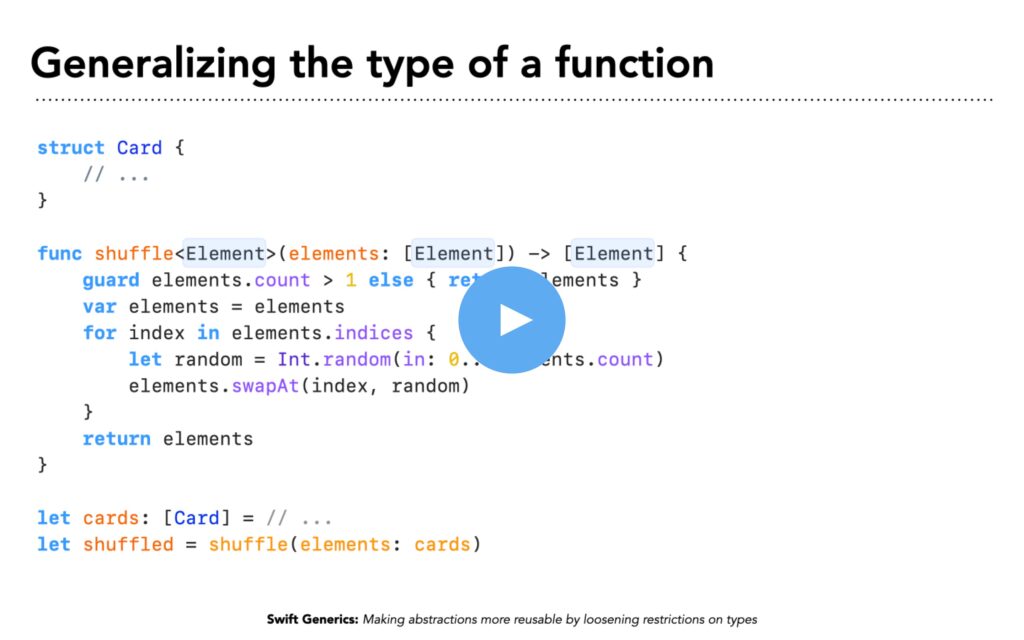
Lesson 2.3 – Swift Generics
Making abstractions more reusable by loosening restrictions on types
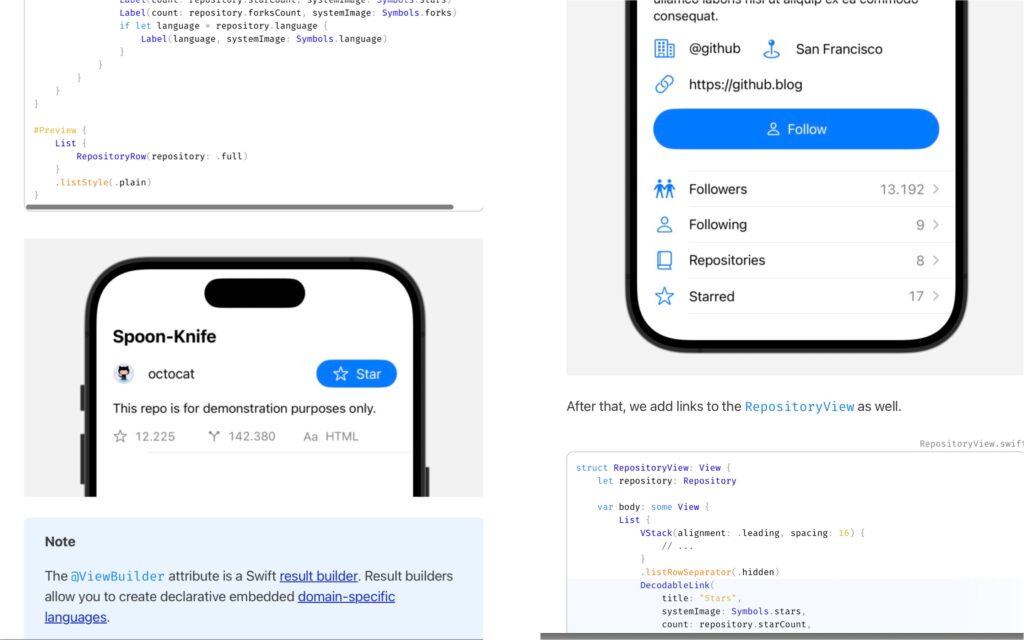
Lesson 2.4 – Generic Views
Reusing complex functionality and reducing code repetition
What you’ll learn:
- Top-down architecture design: how to push high-level requirements down into low-level modules to discover hidden app requirements early during development. Most developers write their code the wrong way.
- Following the Single-responsibility and Dependency Inversion principles in MVC and MVVM to reduce code repetition. Combining these two principles will transform the internal implementation of your SwiftUI views.
- Using Swift generics to overcome the limits of conventional abstractions and share complex functionality across broad SwiftUI views. Plus, how to create custom containers with generic view builders and write robust, expressive, and concise SwiftUI views.
- Implementing typed navigation at the top of the view hierarchy while keeping views decoupled. This creates independent SwiftUI views and a flexible navigation structure.
Module 3
Networking and Architectural Design Patterns
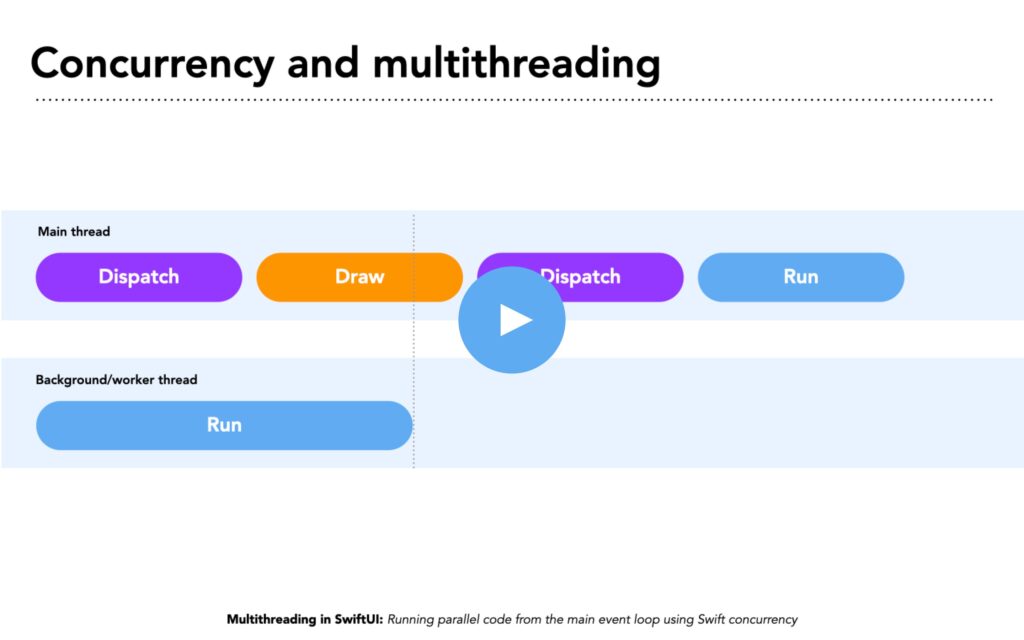
Lesson 3.1 – Multithreading in SwiftUI
Running parallel code from the main event loop using Swift concurrency
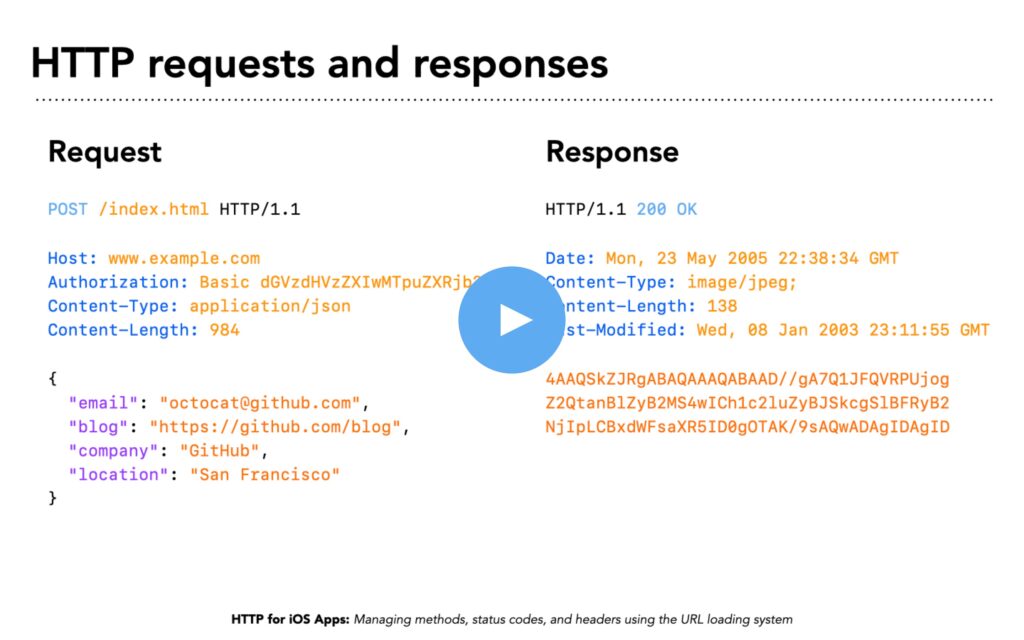
Lesson 3.2 – HTTP and REST for iOS Apps
Managing HTTP methods, status codes, and headers using the URL loading system
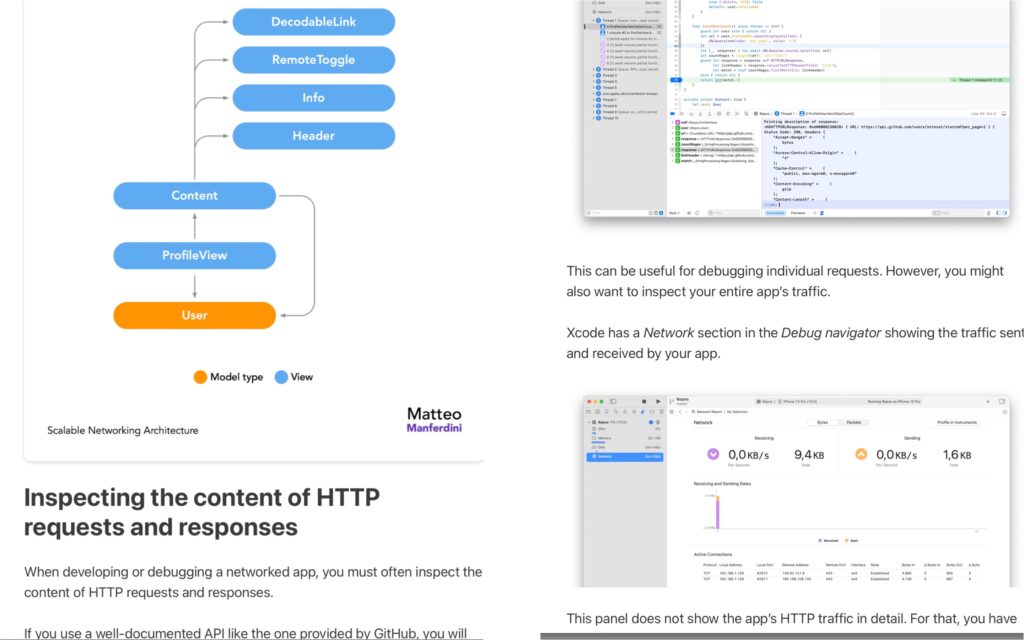
Lesson 3.3 – Massive SwiftUI Views
Performing network requests and separating layout code from view behavior
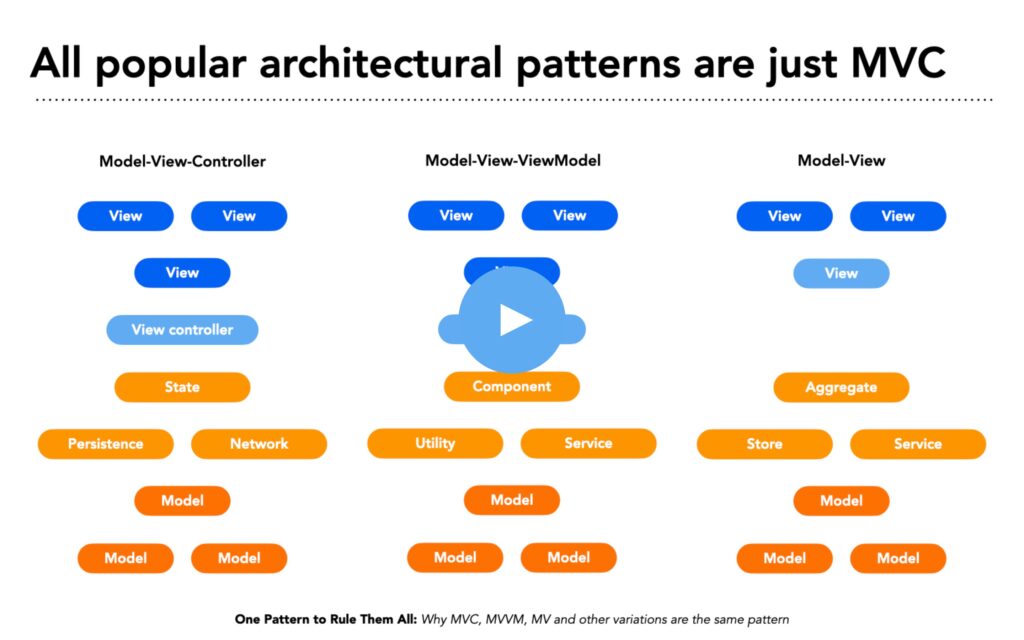
Lesson 3.4 – One Pattern to Rule Them All
Why MVC, MVVM, MV, and other variations are the same pattern
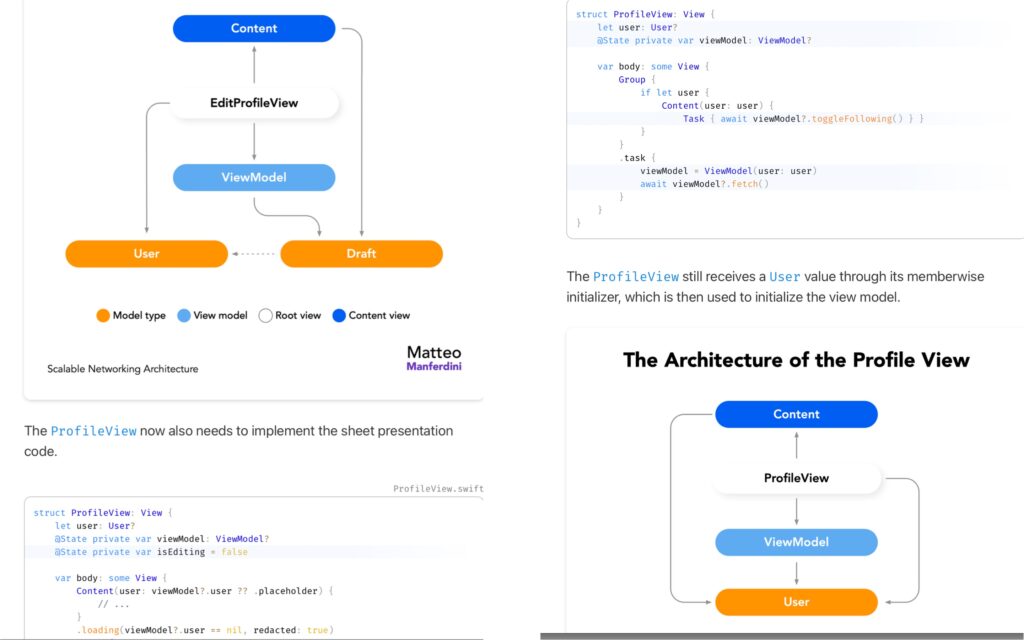
Lesson 3.5 – Navigation and State Transitions
Distributing responsibilities across the view, root, and view model layers
What you’ll learn:
- Why the SwiftUI data flow still follows the original scheme of MVC pattern even decades after its invention. Despite what many claim, all the popular architectural patterns are just variations on MVC, and the MV pattern is nothing but a shortcut.
- Performing HTTP requests through the URL Loading System and handling the status codes returned by REST APIs. Plus, how to implement HTTP caching even for offline Xcode previews that use AsyncImage.
- The main event loop of a SwiftUI app and how multithreading prevents long-running code from freezing the user interface. This is why you need to use asynchronous functions to perform network requests.
- The testability and concurrency issues of placing network requests code inside SwiftUI views. Shortcuts like the MV pattern entirely ignore these issues.
- Why view models are required to remove the tight coupling from massive SwiftUI views. Plus, how to run view model updates on the main thread to avoid data races and satisfy Swift 6’s strict concurrency checking.
Module 4
Complex Authentication Flows and Concurrency
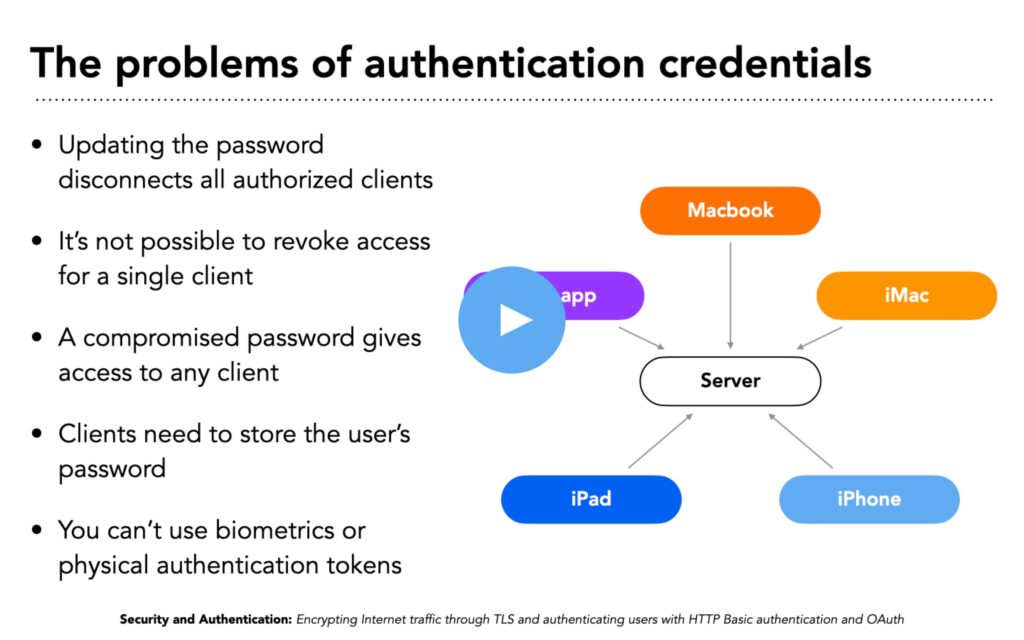
Lesson 4.1 – Security and Authentication
Encrypting Internet traffic through TLS and authenticating users with HTTP Basic authentication and OAuth
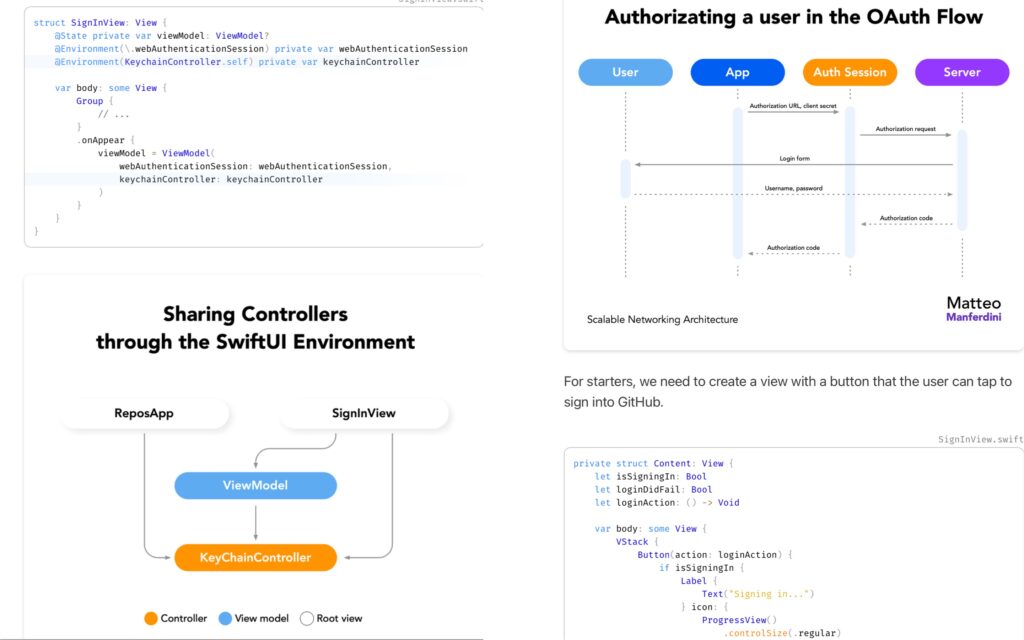
Lesson 4.2 – The OAuth Flow in SwiftUI
Presenting a web authentication session and storing secrets in the Keychain
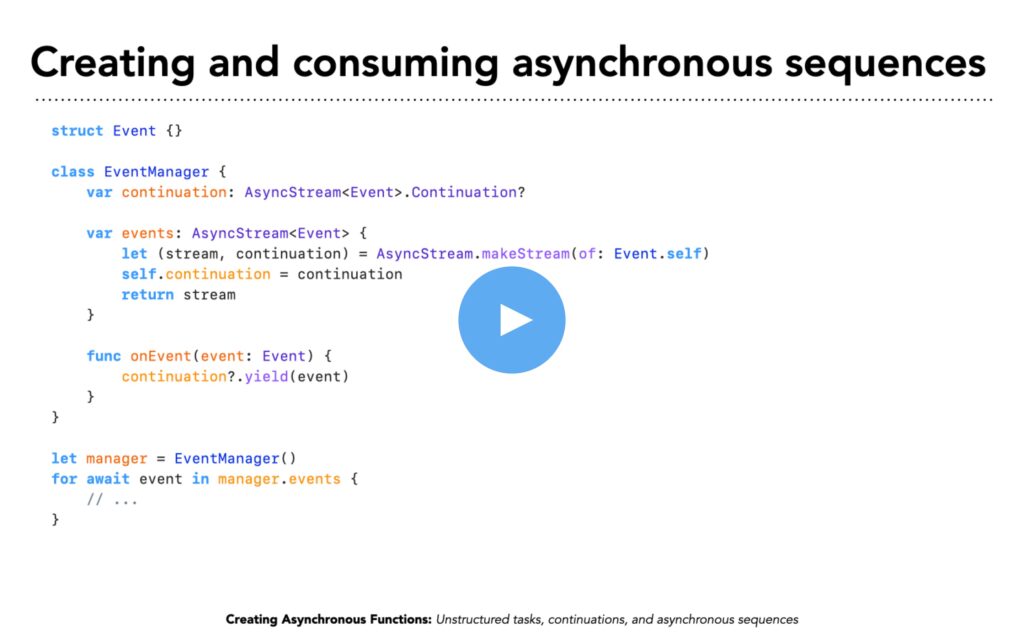
Lesson 4.3 – Creating Asynchronous Functions
Unstructured tasks, continuations, and asynchronous sequences
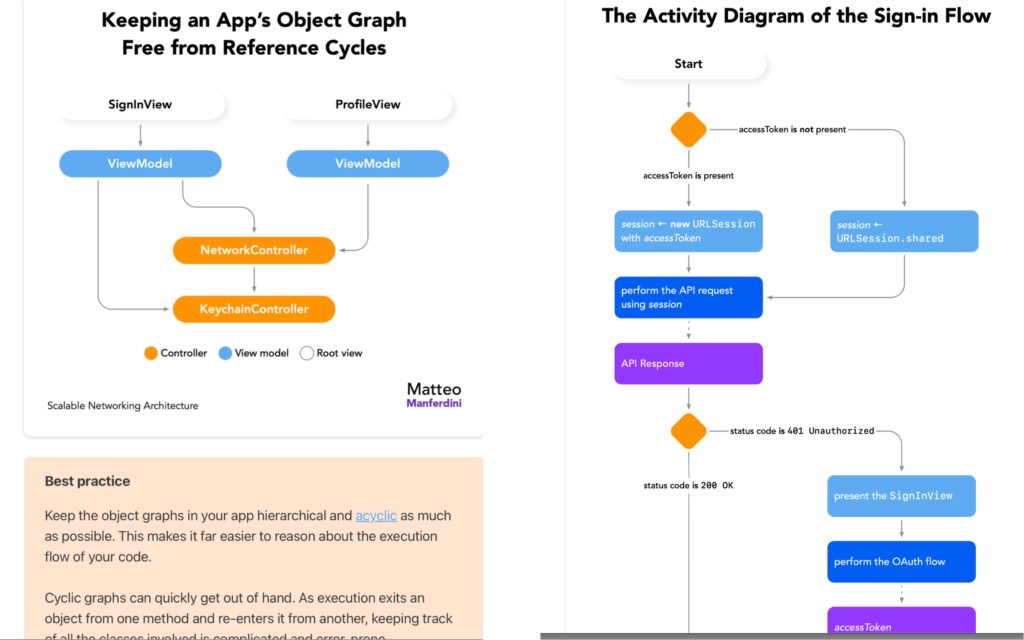
Lesson 4.4 – Implementing the App’s Authentication Flow
Presenting a sign-in view and repeating failed API requests
What you’ll learn:
- Authenticating REST API requests with HTTP basic authentication and OAuth. As an iOS developer, you need to understand how to secure traffic to a server through cryptography and why you can’t securely store your app’s secrets in its binary.
- Storing passwords and authorization tokens in the iOS Keychain without relying on third-party libraries. A keychain controller is essential to keep your app’s networking classes testable.
- How to trigger a complex sign-in flow in a SwiftUI app whenever the server rejects an authenticated request and resume all suspended tasks after authorization. This is a less-known Swift concurrency technique that can streamline your networking code.
Coming soon
Structured Interactive Interfaces with Dynamic Data
- Structured concurrency and actors;
- Protocol-oriented network layer architecture;
- SOLID: the open-closed and interface segregation principles;
- Concurrency problems: data races and priority inversion;
- Handling global state and error handling;
- And more…
A limited offer
This material is very complicated to assemble, so I am still completing it. However, as I have learned from Jay Abraham, I cannot punish my students because of my limited resources. I must share what I know as soon as I can.
The course already has 200+ students who have benefited from the existing material. I am confident that others will also, so I decided to pre-launch the course for Black Friday at a special price.
Scalable Networking Architecture also includes…
Step-by-step analysis of a real-world complex networked app
After theoretical lessons, I apply each concept to the step-by-step implementation of a complex app that deals with the complexities and edge cases you face when developing real-world apps.
No more toy apps that show you only one concept in isolation and leave out the necessary details.
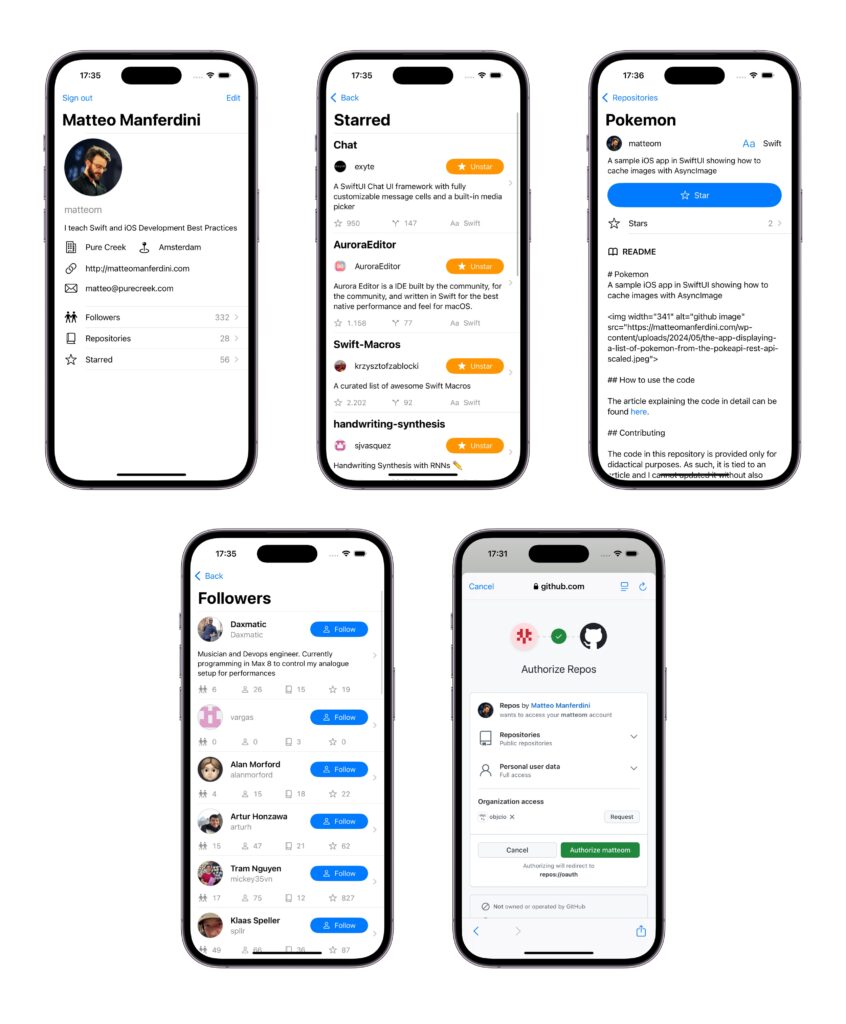
you will see what decisions and considerations go into each app development stage. I will not merely give you the completed code; I will show you how to adapt and refactor it as the app grows, as would happen on an actual software project.
This course provides examples of real solutions that you can apply to your projects. This is possible because the final app will have a user experience that is ready for the App Store.
The Xcode project with the complete Git history to explore the evolution of the app’s code
The material includes the complete Xcode project so you can see the code in context rather than in an isolated lesson.
The project comes with the entire Git history detailing the app’s evolution. This allows you to compare each step to the previous ones and see which code changed and how.
Each commit in the repository has a tag with a lesson number, which helps you quickly return to the proper lesson and refresh the relevant concepts right where they were introduced.
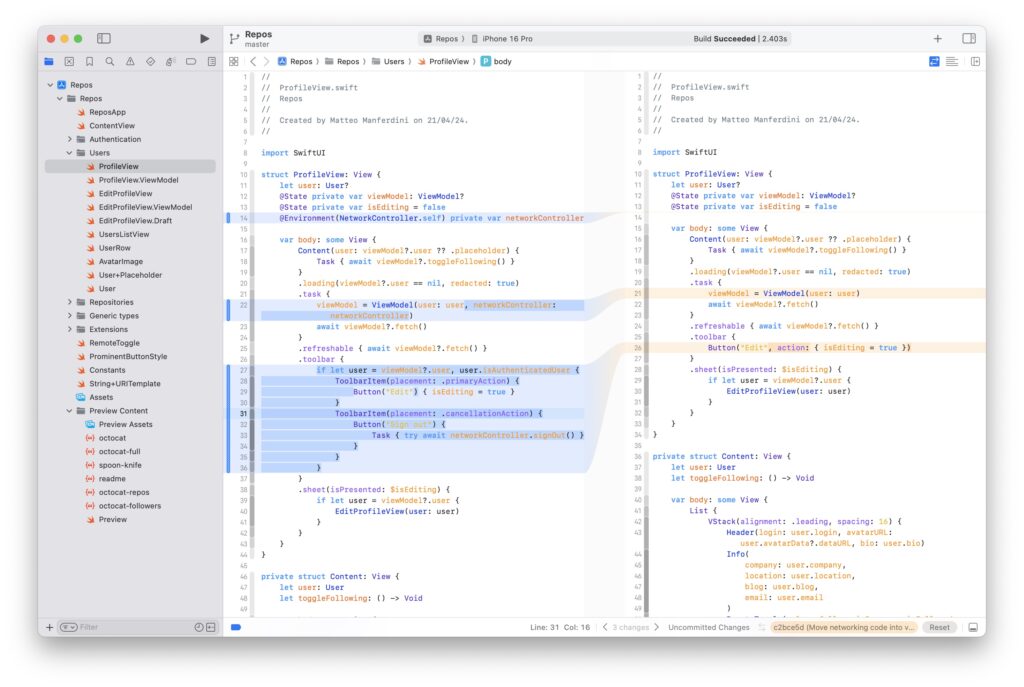
Closed-captioned videos with downloadable slides and transcripts
Each theoretical video lesson has closed captions and speed controls to help you follow the material at your own pace without missing anything.
Video lessons also include downloadable slide decks and transcripts, which allow students to quickly return to the salient parts without sifting through a whole video.
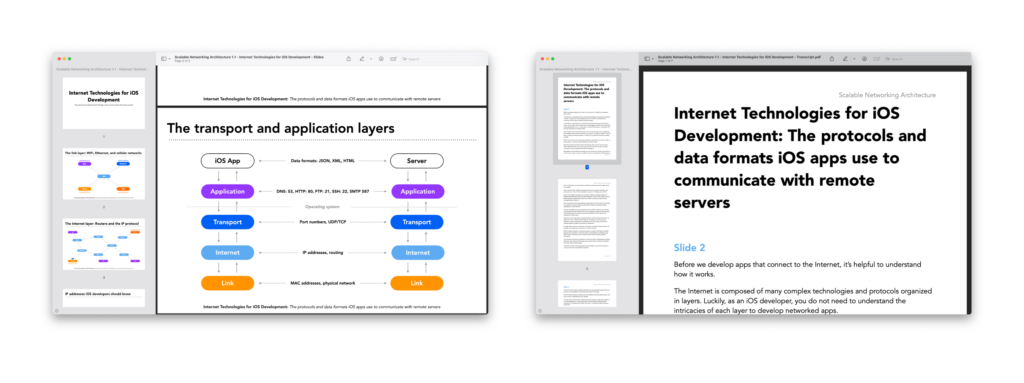
Lifetime access
The course is online and accessible from anywhere. It is pre-recorded, so you don’t have to attend live classes in the middle of your day. You can follow it at your own pace and have lifetime access to the material.
Maybe you need to pause the course to go on vacation, or life and work take over, leaving you with little time. That’s not a problem, though, since you get lifetime access and can resume whenever you want.
You can go over the entire course to have a high-level understanding of each advanced concept as soon as you join. In the future, you can review each lesson to refresh your knowledge and deepen your understanding.
Is Scalable Networking Architecture right for you?
This is an advanced course, so it’s not for everybody.
Scalable Networking Architecture is NOT for you if:
- You are a beginner and don’t know the basic concepts. These include Swift and SwiftUI’s syntax, a basic understanding of structures, classes, and closures, standard views and modifiers, SwiftUI’s property wrappers and data flow, and basic design patterns like MVC. You can find these in my entry-level course, SwiftUI Structural Foundations.
- You are starting from scratch and have never made an app in SwiftUI. While you don’t need a developer job, only experience writing code will help you understand what I discuss in the course.
- You are just looking for code templates to use in your apps and are not serious about learning advanced architectural and software principles. This is not what this course offers. Copy and paste developers, please leave.
- You need to get a job and make money quickly. A course this complex takes time and effort to absorb. Although some students have incredible results in a short time, I cannot guarantee that.
Scalable Networking Architecture is RIGHT for you if:
- You already have experience making iOS apps in SwiftUI and want to take it to the next level.
- You want to explore the advanced concepts behind the architecture of complex networked SwiftUI apps. Instead of random techniques you don’t know how to apply to your apps, you want a complete system.
- You are patient, deliberate, and systematic. You know that advanced topics need to be understood and internalized with time and practice and are not learned overnight.
- You want to learn critical concepts instead of mindlessly repeating the same actions because you don’t know any better.
- You want to understand the big picture of a complex app, not just read specific code pieces to do this or that task.
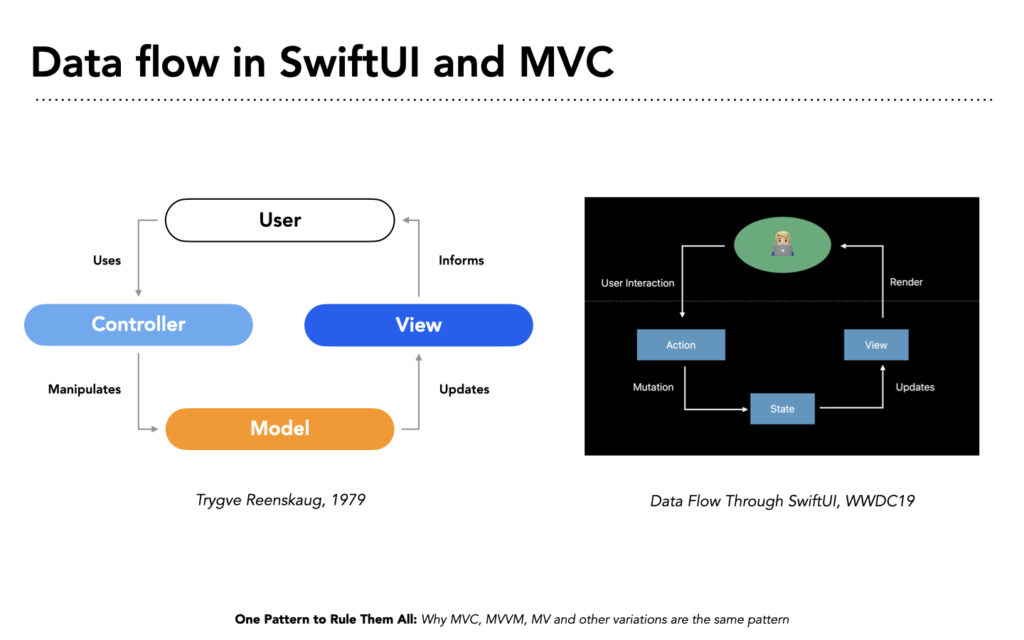
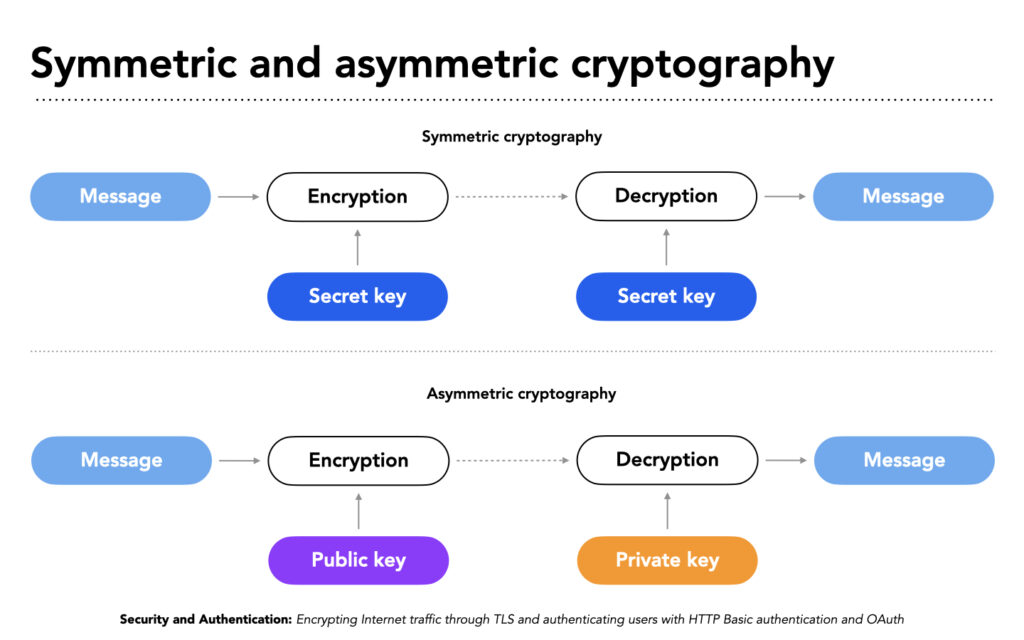
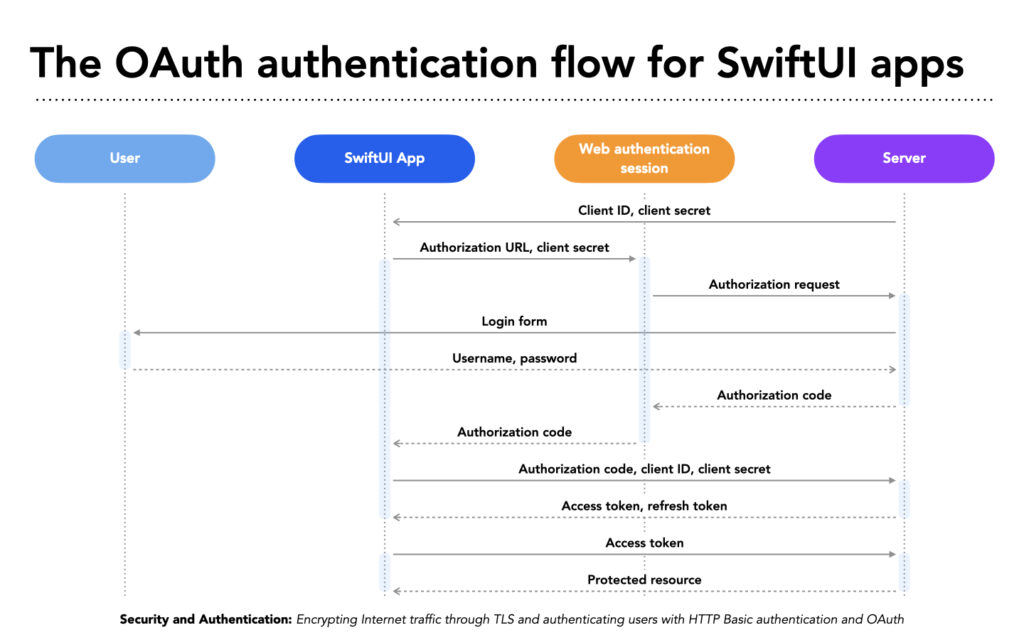
Frequently asked questions
How is Scalable Networking Architecture different from any other course on the market?
This is the only course that focuses on the complete architecture of an app and includes the advanced concepts you need.
There are plenty of books and courses that focus on specific topics. For example, you can learn the ins and outs of Swift concurrency or a particular iOS design pattern, such as MVVM.
But this is the only course that focuses on developing a complex networked app in a coherent system. My students confirmed that there is nothing else on the market like Scalable Networking Architecture.
Why? Such material is challenging to produce and keep up to date, and I seem to be the only one crazy enough to do so.
How is Scalable Networking Architecture different from SwiftUI Structural Foundations?
SwiftUI Structural Foundations is my entry-level course on iOS development with SwiftUI. It covers basic architectural concepts that other courses don’t, but given its nature, it does not explore them deeply.
Moreover, SwiftUI Structural Foundations does not extensively cover networking, REST APIs, and Swift concurrency.
Scalable Networking Architecture comes naturally after SwiftUI Structural Foundations. The two courses have little overlap, and Scalable Networking Architecture assumes you are familiar with the basic concepts covered in SwiftUI Structural Foundations.
You must become familiar with those basic ideas to follow Scalable Networking Architecture. However, you can take the bundle, which includes all my courses, to catch up to the required level.
There seems to be a lot of theory in the course. Is there also practice?
Absolutely. The Scalable Networking Architecture follows my usual approach: first, understand the theory and then apply the concepts to real-world code.
Do you cover the MVVM and MV design patterns?
Yes, but what I cover significantly differs from other online material. I expand concepts and techniques that you cannot find anywhere else.
Moreover, I consider the MV pattern a shortcut, not a legitimate architectural pattern. I mention it only to explain its drawbacks.
Do you cover functional reactive programming (FRP) frameworks like Combine or RxSwift?
No. Despite what some developers claim, these frameworks are just tools and are not required in networked apps.
Moreover, after the introduction of Swift concurrency, the Swift community’s interest moved away from FRP frameworks toward async/await, structured concurrency, async sequences, and async algorithms.
I am also not an expert in FRP; I have never used it and have a natural aversion to it, so I am not the right person to teach it.
Will Scalable Networking Architecture help me if I am a beginner in iOS development and don’t understand SwiftUI architecture well?
Maybe. If you miss the underlying architectural concepts, this course might be too advanced for you, and I recommend that you follow SwiftUI Structural Foundations first.
But if you already have experience making SwiftUI apps and want to take your understanding to the next level, this course is for you. And if you find that this material is above your head, you can always follow my other courses to fill the gaps.
Do I have to commit time every day to follow the course?
No, the program is self-paced, so you can follow it at your own pace. How much time you commit to the course is entirely your choice.
I divided the videos into manageable sizes and text lessons into sections that are always to the point. This allows you to move through the material in discrete steps.
In fact, that’s what I recommend. Biting off more than you can chew can be discouraging.
Even if you proceed slowly, picking one concept at a time can significantly and immediately impact how you write your apps.
The app in the examples is different from the one I am working on. Will Scalable Networking Architecture help me with my specific project?
Most certainly, yes. Every concept in the course is presented in general theory and practical examples.
The concepts I cover will apply to every type of app you work on. Whether you are working on a networked app, a social network app, or a business tool, the architectural concepts in this course always apply to your code.
In fact, I use them in every single app I make.
I can’t think of any app for which you won’t need these fundamental ideas. The only exception might be games, which have their own architecture. Even in that case, most concepts in this course are still valid.
I already have some years of experience in SwiftUI development. Will I benefit from this course?
That is likely. Many of these concepts took me years of research and practice to master. Even if you have worked on SwiftUI for some time, you might not have seen them applied in a real app.
The people you work with significantly impact the number of ideas you are exposed to. Of course, there is a chance that you are advanced enough to know most of the concepts already, which is why I offer a 60-day money-back guarantee.
I am afraid that this course might be too introductory. Is it really an advanced course?
Yes, it is. I poured all the knowledge I gathered through years of experience and research into this program.
I worked with many developers from different backgrounds and studied other languages. I also tested and expanded each concept before introducing it in each lesson. Finally, I developed some unique approaches, which you will find only here.
Rest assured that you must be a pretty advanced developer not to find any new ideas in this course. If that is the case, I have a 60-day money-back guarantee, so you might as well try it and see for yourself.
Do I need a Mac Pro or a MacBook Pro for this course?
No. The concepts in this course are about how to write code correctly and architect apps accurately. They are advanced on a conceptual level, not computationally complex.
Any Mac computer that runs the latest version of Xcode is enough.
Can I find this information in cheaper courses on the market?
Not in a single course. You can find good material on specific topics if you are looking for cheaper solutions. However, in my experience, they usually leave out many real-life development problems and practices.
Moreover, you must spend a lot of time putting all the concepts together yourself.
Even without considering this massive time investment, if you were to add together the prices of alternative books and courses to learn everything I cover in this course, their cost would be comparable.
When considering pricing, consider how much your time is worth. I believe that my course offers a higher return on investment than other material on the market. I offer monthly payment plans if you prefer to pay in smaller amounts instead of a lump sum.
Of course, you might disagree, so I offer a 60-day money-back guarantee.
Can I join the course only for a few months and then cancel my subscription?
No. The payment plans are only a way to spread the payments over several months and help those who cannot commit the entire amount at once.
These installments are not a subscription service you can cancel anytime, as you find on other websites. If you select a payment plan, you must complete all installments.
Of course, my 60-day money-back guarantee still applies, regardless of your payment option.
Yes, you have other options to learn these concepts
I already told you that Scalable Networking Architecture includes a complete system that covers all the advanced concepts you need and shows you their relationship.
That said, I will not lie and tell you that you can learn these concepts only here. You can also find them elsewhere (excluding the few unique techniques I developed).
Here is what it took me to put together all this information into a coherent system:
- A bachelor’s degree in computer science and a master’s degree in computational logic;
- Almost 20 years of experience in different programming languages, frameworks, and projects, working for several companies and teams, both as an employee and as a freelancer;
- Years of research with online articles, books, Apple’s documentation, and WWDC videos;
- Thousands of hours of experimentation with code both in my published apps and during the creation of this course.
If you want, you can do the same too.
You have other options as well. Maybe you have even tried some of them already:
Attend iOS Boot Camps, retreats, corporate training, or online coaching. These usually cost between $3,000 and $20,000.
Some can be excellent, but I have met many people who attended them and did not even learn the concepts I cover in my introductory courses, let alone any of the material in Scalable Networking Architecture.
Get the required experience on the job: You can learn a lot working as an iOS developer in some companies and interacting with other developers.
If you join a top company, you can get experience and get paid for it! Unfortunately, at most jobs, you might be the only developer with no one to learn from, or you might work in a company where most of the developers in the team just want to do their job and go home.
You might also be the one that knows more than others. You might even face resistance from colleagues when you want to try new approaches.
Learn all the concepts from books, online articles, and open-source projects. I did a lot of this myself.
I subscribe to all iOS newsletters, watch videos from Apple and other conferences constantly, and read many books on advanced Swift, unit testing, advanced networking, etc.
I even studied functional languages like Lisp, Clojure, and Haskell. That is why it took me almost 20 years to put together all this knowledge.
You can do that, too, but why would you?
Do nothing: yes, you have this choice, too, and it costs no money.
You can keep doing your job as you know it, making the same mistakes again and fixing problem after problem. How much time can you go on like that?
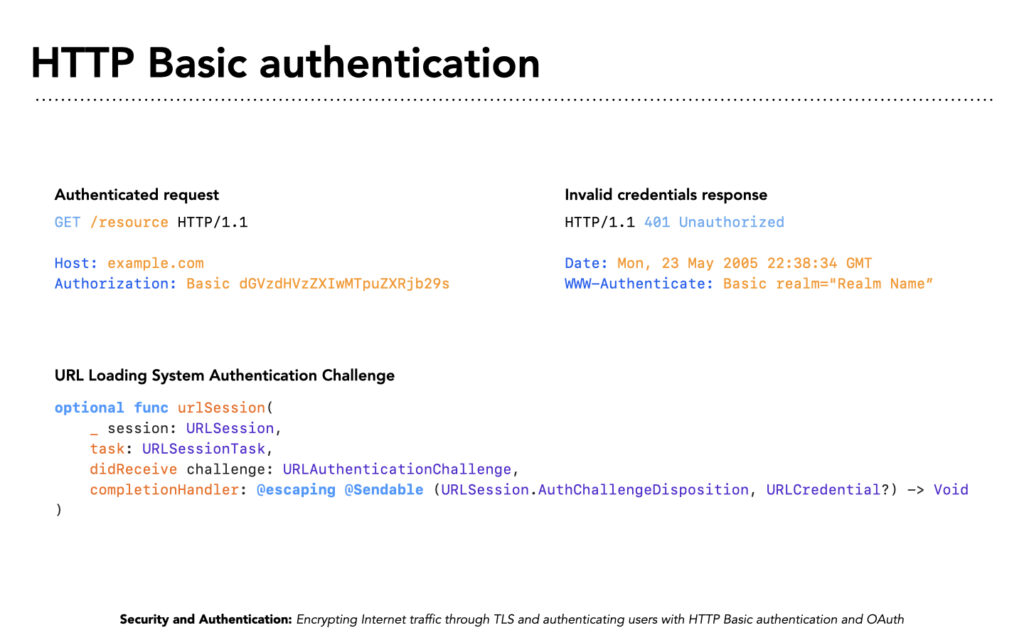
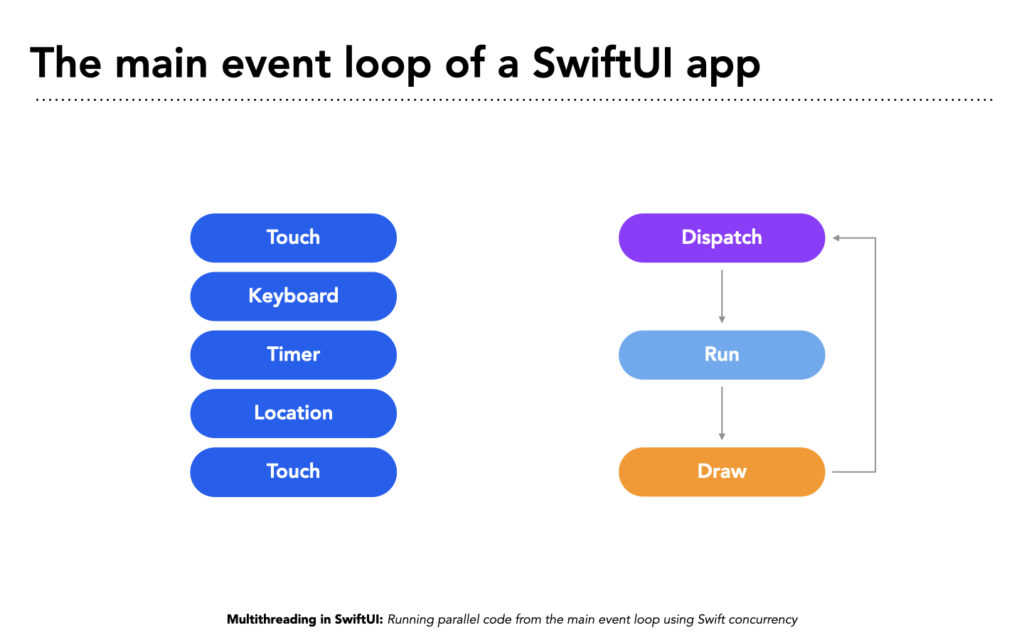
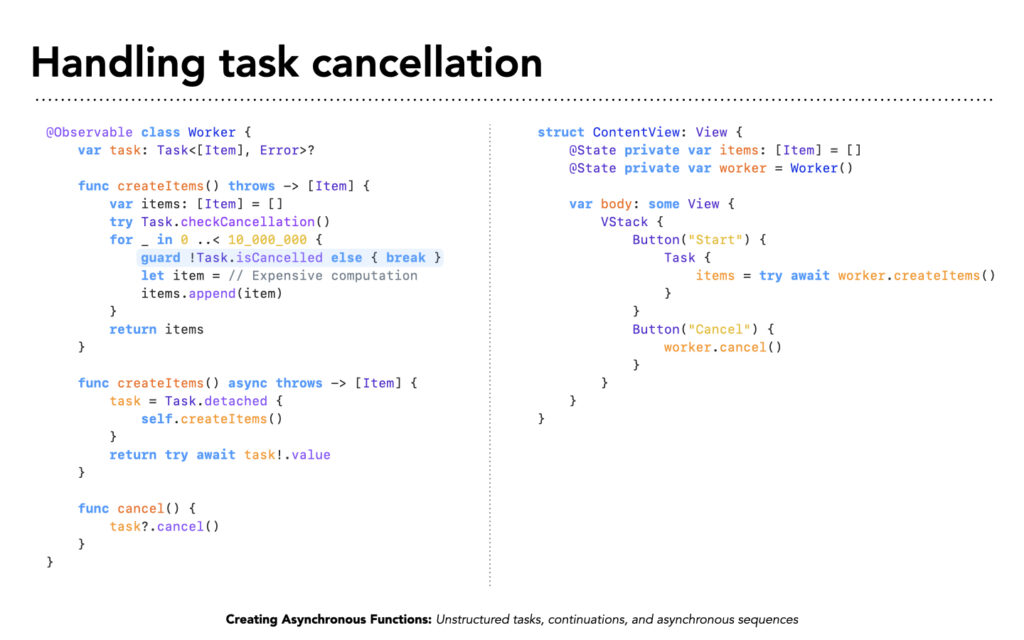
An unbeatable guarantee: try Scalable Networking Architecture for a full 60 days, BETTER than 100% risk-free
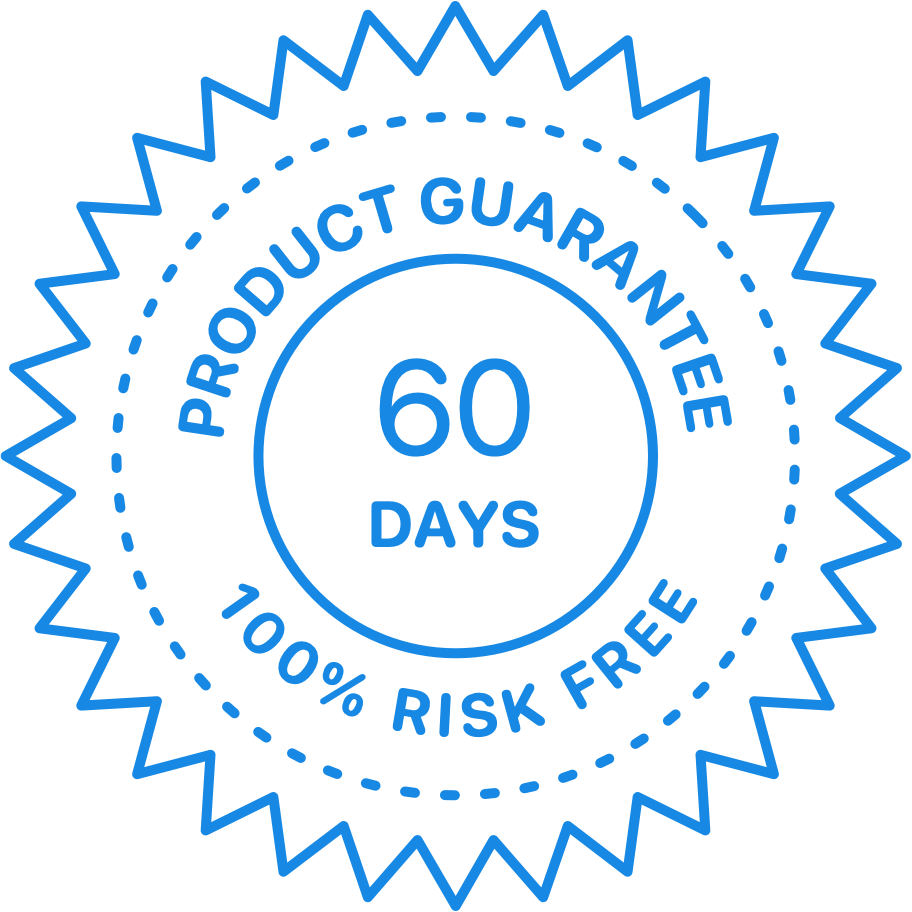
Try the entire Scalable Networking Architecture. If it doesn’t help you build advanced iOS apps, I insist you get 100% of your money back. In addition, I will buy you any book or course of your choice from my competitors, up to $200 in value.
Let me tell you why I offer this unbeatable guarantee.
I tried many other books and courses and watched countless talks, but none covered these concepts. I had to disassemble all the ideas to build a coherent system for making networked iOS apps.
I often buy “advanced” books on specific topics, like unit testing or networking, only to be disappointed. These books build a trivial app, show you a collection of tactics, and then leave you to figure out the rest yourself.
None of these books ever considered the architecture of a real app. Most of these books focus on beginners or give simple solutions you cannot apply to your work. I am honestly tired of these books. Software development does not happen with copy-and-paste. You need to understand all the implications of what you do to decide on the best solution for your app.
It took me years to organize and assemble this material in my previous flagship course in a coherent system that progresses logically and allows you to build concepts upon one another.
It took several months to reorganize it in this course, built explicitly for SwiftUI and Swift Concurrency.
I know this material works. It includes much I have learned and used in almost twenty years of development on Apple platforms, and I have discussed these techniques with many other developers.
I also tested the course with different students, and they got massive value. And I know there is nothing else like it on the market.
That’s why I more than guarantee Scalable Networking Architecture.
Are there any catches to my offer? Not at all, but there is a straightforward stipulation. I’m sure you’ll agree that it’s pretty reasonable.
My students have shown me, without a doubt, the enormous potential my course produces and how it allows you to move forward in massive strides. One of my students even tripled his salary two months after joining.
However, I don’t know if you’ll study the material as I structured it and apply the techniques I explain to your work. So, I ask that you agree to follow through and document your actions.
If you want to join the course because you are curious, coast along, check lessons at random, or even leave the course aside to gather dust, that is your prerogative. Your time and money are yours to dispose of as you please. But I cannot take responsibility for your inaction.
Take the course and see for yourself.If it does not work, email me and prove that you made a good-faith attempt to follow it. I will refund 100% of your money and buy you one book or course of your choice from one of my competitors, up to $200 in value.
This guarantee extends for 60 days after joining, enough to go through the ENTIRE course.
If you don’t love it, email me anytime during the first 60 days and show me you’re applying the material, but it does not work for you.
By the way, I ask you to show me that you’ve applied the concepts of the course because I know how powerful they can be when used.
I have no intention of keeping your money if you’re dissatisfied, but I insist that you commit to taking action if you want to get results from this program. Reading a few lessons and doing the same things you did before will not be enough.
I’ve spent years learning these concepts, researching, and creating this unique material. If you’re ready to build more complex, maintainable, testable, and scalable iOS apps, I’d like to share it with you.
Which option is right for you?
Option #1
Scalable Networking Architecture
- Scalable Networking Architecture (
$799$499): The complete SwiftUI networking course for iOS developers who feel stuck and want to go past simple online tutorials for beginners, moving from intermediate to expert. SwiftUI Structural Foundations ($299): The practical course to build a solid understanding of SwiftUI foundations, enabling you to create any iOS app confidently. SwiftUI Structural Foundations is an online course focusing on the best patterns and practices for structuring the code of fully featured iOS apps.Git for iOS Development ($79): The practical course to fully use Git, safeguard your Xcode projects, and share code with other iOS developers without being frightened by its arcane-sounding commands. Git can be remarkably difficult to use if you get the wrong mental model taught by many “Getting Started” guides that only teach basic commands. Git for iOS Development covers everything you need to know about Git in Xcode and the Terminal.The SwiftUI Compendium ($49): The missing SwiftUI manual designed for aspiring iOS developers seeking to understand the basics of SwiftUI through a practical project, leveraging Apple’s official documentation. The SwiftUI Compendium is a concise quick-start guide without unnecessary details to build a foundation from which you can expand your knowledge and skills.Total value: $926. This course bundle lets you save $127 on the total price when buying all the courses separately.
Pre-launch price
7 monthly payments of $139 $89
(That’s only $2.97 per day. Sales taxes may apply depending on your location)

If you have any questions or concerns about the course, email me. I am happy to help. Team/bulk discounts are also available.
Option #2
The Ultimate iOS Professional Bundle
- Scalable Networking Architecture (
$799$499): The complete SwiftUI networking course for iOS developers who feel stuck and want to go past simple online tutorials for beginners, moving from intermediate to expert. - SwiftUI Structural Foundations ($299): The practical course to build a solid understanding of SwiftUI foundations, enabling you to create any iOS app confidently. SwiftUI Structural Foundations is an online course focusing on the best patterns and practices for structuring the code of fully featured iOS apps.
- Git for iOS Development ($79): The practical course to fully use Git, safeguard your Xcode projects, and share code with other iOS developers without being frightened by its arcane-sounding commands. Git can be remarkably difficult to use if you get the wrong mental model taught by many “Getting Started” guides that only teach basic commands. Git for iOS Development covers everything you need to know about Git in Xcode and the Terminal.
- The SwiftUI Compendium ($49): The missing SwiftUI manual designed for aspiring iOS developers seeking to understand the basics of SwiftUI through a practical project, leveraging Apple’s official documentation. The SwiftUI Compendium is a concise quick-start guide without unnecessary details to build a foundation from which you can expand your knowledge and skills.
- Total value: $926. This course bundle lets you save $127 on the total price when buying all the courses separately.
Pre-launch price
7 monthly payments of $179 $139
(That’s only $4.63 per day. Sales taxes may apply depending on your location)

Pay now in full and save another $174
If you have any questions or concerns about the course, email me. I am happy to help. Team/bulk discounts are also available.
Learning how to make complex networked apps is not an accident.
You can get to the point where you can confidently build a simple SwiftUI app and ship it to the App Store. That is not even an easy task by itself. It’s an accomplishment that many fail to reach.
But it only gets you that far.
When an internet-connected app project grows in complexity, it becomes less and less evident how you should organize its code. You keep adding new network requests to existing classes because it seems natural until they become unmanageable and untestable monsters.
The kind of experience you need to make scalable networked apps and write reliable code takes time to accumulate. You need to spend significant time working on big projects with experienced developers from which you can learn.
But those are rare and hard to find. If you want to become one yourself, what paths have you left?
Specific books can help. I read many of them and learned valuable lessons. However, those lessons were scattered across many books that took me ages to read—not counting the books that provided no helpful insight.
And I still had to put all these ideas together myself.
You can do that too. If you are the kind of person who enjoys the painstaking process of putting together all the pieces of the puzzle, go for it. You will have enough to keep yourself busy for a long time.
Take a shortcut to mastery
I don’t know you, but I prefer to reach my goals as soon as possible. I don’t care about putting the information together all by myself.
I want to make apps, not discover what others have already found.
Unfortunately, I didn’t have such a choice. I wish I had this course when I started. Instead, I had to go through 20 years of experience and research to build my current knowledge.
But now, you have a choice.
You can get there in a fraction of the time that cost me.
Spending only a few hours per week, you can start writing better code in your apps. This will not only affect your networking skills but will significantly impact any type of app you develop.
You can gain insights that took me years to discover in a few months. These are timeless principles that you will use throughout your career as a developer. A student of mine tripled his salary two months after joining a previous version of this course.
Where will this lead you in a few years?
You can get much further in your career, ship sophisticated apps to the App Store, and get more satisfaction from your work. I don’t know how this could impact your life, but you do.
My mentors taught me three critical principles that allowed me to propel my life forward faster than I could ever do alone. I give them all the credit for teaching me these lessons, but I also know I implemented them myself.
You can do that too.
Do what other developers won’t do
Most developers follow a few tutorials, copy and paste code from Stack Overflow, and spend their whole careers making the same mistakes again and again. I’ve grown tired of fixing messed-up projects created by agencies or freelancers.
That approach works for one-shot apps left to rot in the App Store after publication. Apple brags about the 2 million apps in the App Store, but we know that most are garbage.
For better or for worse, these low-quality developers will continue to exist. There is always a demand for cheap and quick app development.
Is that the kind of work you want to produce?
You can surpass these people by focusing and working hard to learn the high-level concepts of complex SwiftUI architectures.
That is harder than copying and pasting code from tutorials. But that’s good. Since most people won’t go past such a low bar, doing otherwise will put you immediately in front of everybody else.
Give yourself every advantage
When I started making apps, I had to find all the information myself. That led to a problem: I kept the same mentality for too long.
When I became skilled enough to make apps, I gained enough knowledge to be dangerous. I released app after app that failed miserably in the App Store.
When I later decided to become a freelancer, I almost went bankrupt before I learned how to run a business properly.
Only when I got serious and stopped trying to figure everything out by myself did I leap forward. To this day, I have spent tens of thousands of dollars on courses and coaching because they give me a tremendous advantage over everyone else.
It’s for that exact reason that I am here teaching you what I learned.
Learn from the best
In every human endeavor, a few people achieve the most results. This is called the Pareto Principle: 20% of the people get 80% of the rewards.
This is because these people have more experience and training than others. They have seen more novel situations, and their accumulated knowledge has a powerful compounding effect that puts them at the top.
That is why I now learn from the best in every field, even if I have to pay 100x the money I would spend on a few books.
If you want to make complex networked apps, you can buy, for starters, a book on SOLID principles, a book on Swift concurrency, a book on networking, and a book on advanced Swift techniques. Each of these books will only cost you 10 to 30 bucks.
Or you could learn from someone who has already read all those books and many others, worked on complex projects for big companies, and created several complex apps still used by thousands.
I can’t say I am the best. As the saying goes, “You can’t read the label from inside the jar.” But you can. If you are reading this, you have followed me long enough to assess the quality of my work.
Who would you rather learn from?
Jump ahead instead of trying random tactics that give you no results
I believe the material I compiled for this course will propel you forward in ways no other material on the market can. The students who joined this course have already told me so, so I know this material is solid.
If you want to create apps with beautiful architecture that will stand the test of time, you can do it much faster than you think. It’s not just about the money that can come from it. We all do it for ourselves, to take pride and satisfaction in the code we create.
It’s time to make a decision.
Scalable Networking Architecture will soon close. When I close my courses, I often don’t reopen them for months. So, if you want to create complex networked apps in SwiftUI, you must act now.
Even at this uncompleted stage, the course offers massive value. More than 200 students have already done so, so I am offering it to you now at a lower price.
I also protect you with a 60-day money-back guarantee to make your decision risk-free.
A year from now, you’ll be a year older. What are you going to do?
Option #1
Scalable Networking Architecture
- Scalable Networking Architecture (
$799$499): The complete SwiftUI networking course for iOS developers who feel stuck and want to go past simple online tutorials for beginners, moving from intermediate to expert. SwiftUI Structural Foundations ($299): The practical course to build a solid understanding of SwiftUI foundations, enabling you to create any iOS app confidently. SwiftUI Structural Foundations is an online course focusing on the best patterns and practices for structuring the code of fully featured iOS apps.Git for iOS Development ($79): The practical course to fully use Git, safeguard your Xcode projects, and share code with other iOS developers without being frightened by its arcane-sounding commands. Git can be remarkably difficult to use if you get the wrong mental model taught by many “Getting Started” guides that only teach basic commands. Git for iOS Development covers everything you need to know about Git in Xcode and the Terminal.The SwiftUI Compendium ($49): The missing SwiftUI manual designed for aspiring iOS developers seeking to understand the basics of SwiftUI through a practical project, leveraging Apple’s official documentation. The SwiftUI Compendium is a concise quick-start guide without unnecessary details to build a foundation from which you can expand your knowledge and skills.Total value: $926. This course bundle lets you save $127 on the total price when buying all the courses separately.
Pre-launch price
7 monthly payments of $139 $89
(That’s only $2.97 per day. Sales taxes may apply depending on your location)

If you have any questions or concerns about the course, email me. I am happy to help. Team/bulk discounts are also available.
Option #2
The Ultimate iOS Professional Bundle
- Scalable Networking Architecture (
$799$499): The complete SwiftUI networking course for iOS developers who feel stuck and want to go past simple online tutorials for beginners, moving from intermediate to expert. - SwiftUI Structural Foundations ($299): The practical course to build a solid understanding of SwiftUI foundations, enabling you to create any iOS app confidently. SwiftUI Structural Foundations is an online course focusing on the best patterns and practices for structuring the code of fully featured iOS apps.
- Git for iOS Development ($79): The practical course to fully use Git, safeguard your Xcode projects, and share code with other iOS developers without being frightened by its arcane-sounding commands. Git can be remarkably difficult to use if you get the wrong mental model taught by many “Getting Started” guides that only teach basic commands. Git for iOS Development covers everything you need to know about Git in Xcode and the Terminal.
- The SwiftUI Compendium ($49): The missing SwiftUI manual designed for aspiring iOS developers seeking to understand the basics of SwiftUI through a practical project, leveraging Apple’s official documentation. The SwiftUI Compendium is a concise quick-start guide without unnecessary details to build a foundation from which you can expand your knowledge and skills.
- Total value: $926. This course bundle lets you save $127 on the total price when buying all the courses separately.
Pre-launch price
7 monthly payments of $179 $139
(That’s only $4.63 per day. Sales taxes may apply depending on your location)

Pay now in full and save another $174
If you have any questions or concerns about the course, email me. I am happy to help. Team/bulk discounts are also available.